python playwright获取二进制文本
时间: 2023-10-13 07:05:44 浏览: 160
要获取二进制文本,您需要使用Playwright的`page.content()`方法并设置`binary`选项为`True`,这将返回一个`bytes`类型的对象,而不是默认的`str`类型。以下是一个简单的示例代码:
```python
import asyncio
from playwright.async_api import async_playwright
async def main():
async with async_playwright() as p:
browser = await p.chromium.launch()
page = await browser.new_page()
await page.goto('https://example.com')
content = await page.content(binary=True)
print(content)
asyncio.run(main())
```
在上面的代码中,我们通过将`binary`选项设置为`True`来获取页面内容的二进制表示。在这种情况下,`content`变量将包含以字节形式编码的HTML代码。
相关问题
python playwright 获取当前url
要使用 Python Playwright 获取当前 URL,可以使用以下代码:
```python
import asyncio
from playwright.async_api import async_playwright
async def get_current_url():
async with async_playwright() as p:
browser = await p.chromium.launch()
page = await browser.new_page()
await page.goto('https://www.example.com')
current_url = page.url
await browser.close()
return current_url
# 使用 asyncio 运行异步函数
current_url = asyncio.run(get_current_url())
print(current_url)
```
这个例子中,我们使用了一个异步函数 `get_current_url` 来获取当前 URL。在函数中,我们使用 Playwright 启动了一个 Chromium 浏览器,并在新页面中打开了一个网址。然后我们使用 `page.url` 获取当前 URL,并关闭了浏览器。最后,我们返回了获取到的 URL。
python playwright库获取元素链接
要获取元素链接,您可以使用 Playwright 库中的 `element_handle.get_attribute()` 方法获取元素的 `href` 属性。下面是一个示例代码:
```python
from playwright.sync_api import Playwright, sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto('https://www.example.com')
link_element = page.query_selector('a')
link_href = link_element.get_attribute('href')
print(link_href)
browser.close()
```
在这个示例中,我们首先使用 `query_selector()` 方法获取一个链接元素,然后使用 `get_attribute()` 方法获取该链接元素的 `href` 属性,并将其打印出来。
阅读全文
相关推荐
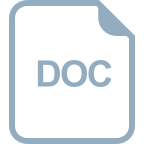
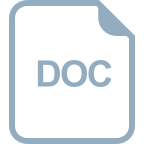
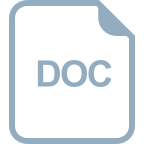













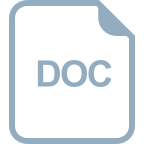