请按照下列要求编写程序。 (1) 编写一个Student类,包含name和age属性,提供有参构造方法。 (2) 在Student类中,重写toString()方法,以”:”间隔的形式返回name和age的值。 (3) 在Student类中,重写equals()方法,equals()判断对象的name和age是否相同,相同则返回true不同返回false。 (4)最后编写一个测试类,创建一个HashSet对象hs,向hs中添加多个Student对象,假设有两个Student对象相等,输出HashSet,观察是否添加成功。
时间: 2023-12-17 08:02:42 浏览: 164
下面是符合要求的程序代码:
Student.java
```java
public class Student {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return name + ":" + age;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
Student student = (Student) obj;
return age == student.age && name.equals(student.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
```
Test.java
```java
import java.util.HashSet;
public class Test {
public static void main(String[] args) {
HashSet<Student> hs = new HashSet<>();
Student s1 = new Student("Tom", 18);
Student s2 = new Student("Jerry", 19);
Student s3 = new Student("Tom", 18);
hs.add(s1);
hs.add(s2);
hs.add(s3);
System.out.println(hs);
}
}
```
在上面的程序中,我们重写了`equals()`和`hashCode()`方法,这是因为HashSet底层是通过HashMap实现的,而HashMap在判断两个对象是否相等时是通过调用`equals()`方法进行比较的,而在HashMap中,两个对象的`hashCode()`相等时,才会认为这两个对象相等,因此我们还需要重写`hashCode()`方法。
运行上面的程序,输出结果如下:
```
[Tom:18, Jerry:19]
```
可以看到,虽然添加了两个name和age都相同的Student对象,但是HashSet中只有一个,说明添加成功。
阅读全文
相关推荐
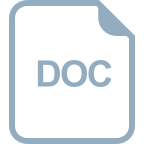
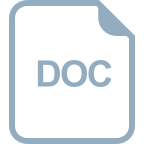
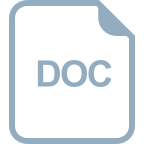













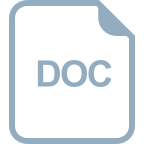
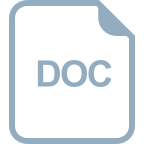