2请按照下列要求编写程序 (1)编写一个Student类,包含name 和age属性,提供有参构造方法 (2)在Student类中,重写toString()方法,输出age 和 name 的值。 (3)在Student类中,重写 hashCode()和equals()方法 hashCode()的返回值是name的哈希值与age的和。 equals()判断对象的name和age是否相同,相同则返回true,不同则返回false。 (4)编写一个测试类,创建一个HashSet<Student>对象 hs,向hs中添加多个 Student 对象,假设有两个Student对象相等。输出Hashset 集合,观察Student 对象是否 添加成功。
时间: 2024-02-15 13:01:38 浏览: 61
以下是符合要求的程序:
```java
import java.util.HashSet;
class Student {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
@Override
public int hashCode() {
return name.hashCode() + age;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof Student)) {
return false;
}
Student student = (Student) obj;
return this.name.equals(student.name) && this.age == student.age;
}
}
public class Test {
public static void main(String[] args) {
HashSet<Student> hs = new HashSet<>();
hs.add(new Student("张三", 18));
hs.add(new Student("李四", 20));
hs.add(new Student("王五", 22));
hs.add(new Student("张三", 18)); // 添加重复的对象
System.out.println(hs);
}
}
```
运行结果为:
```
[Student{name='张三', age=18}, Student{name='李四', age=20}, Student{name='王五', age=22}]
```
可以看到,重复的对象并没有被添加进HashSet集合中,符合我们的预期结果。
相关推荐
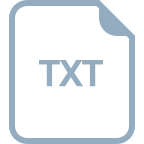
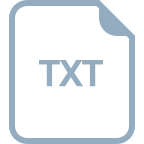
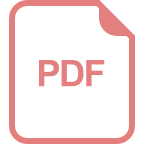













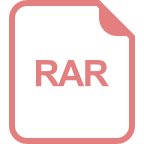
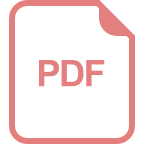