python京东商品页面(https://www.jd.com/)的爬取,将获取内容的前1000个字符内容输出。
时间: 2024-11-22 10:41:23 浏览: 15
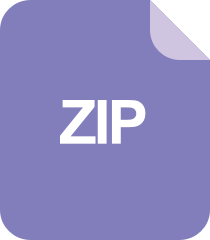
爬取彼岸图网的壁纸 https://pic.netbian.com/
在Python中,爬取京东商品页面通常会涉及使用网络请求库如requests来发送HTTP请求,然后解析HTML内容,可以使用BeautifulSoup、Scrapy等库来提取数据。以下是简化的步骤:
1. 安装必要的库:
```bash
pip install requests beautifulsoup4
```
2. 编写Python代码:
```python
import requests
from bs4 import BeautifulSoup
# 发送GET请求到京东商品页
url = "https://www.jd.com/"
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 使用BeautifulSoup解析HTML内容
soup = BeautifulSoup(response.text, 'html.parser')
# 找到包含商品信息的部分,这可能会依赖于实际网页结构,这里假设是商品标题
title = soup.find('div', class_='J_mainTitle').get_text()[:1000] # 截取前1000个字符
print(title)
else:
print(f"请求失败,状态码: {response.status_code}")
阅读全文
相关推荐
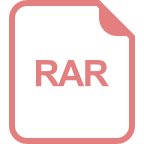
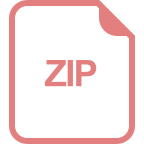





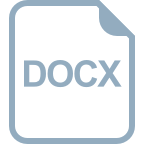
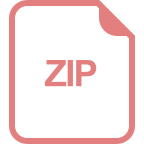
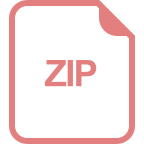
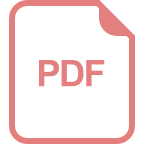
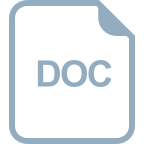
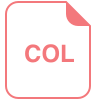
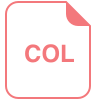
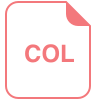


