js代码开发一个修改密码表单界面,包含3个表单项:旧密码、新密码和确认密码,监听表单提交事件,当表单提交时完成如下3种检查:1、要求输入的旧密码字符个数必须大于6个字符小于12个字符,否则在旧密码输入框后面以红色字体提示“旧密码长度有误”;2、要求输入的新密码和确认密码相同,否则在确认密码输入框后面以红色字体显示“新密码与确认密码不一致”;3、新密码里必须包含数字、字母和特殊符号,否则在输入新密码提示框后面提示“必须包含数字、字母和特殊符号”
时间: 2024-01-22 09:17:51 浏览: 208
```html
<!DOCTYPE html>
<html>
<head>
<title>修改密码表单</title>
<meta charset="utf-8">
<style type="text/css">
form {
width: 300px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
}
input {
display: block;
margin-bottom: 10px;
padding: 5px;
border-radius: 5px;
border: 1px solid #ccc;
width: 100%;
}
label {
display: block;
margin-bottom: 5px;
font-weight: bold;
}
.error {
color: red;
font-size: 12px;
margin-top: 5px;
}
</style>
</head>
<body>
<form id="password-form">
<label for="old-password">旧密码:</label>
<input type="password" id="old-password" name="old-password">
<label for="new-password">新密码:</label>
<input type="password" id="new-password" name="new-password">
<label for="confirm-password">确认密码:</label>
<input type="password" id="confirm-password" name="confirm-password">
<button type="submit">提交</button>
</form>
<script type="text/javascript">
var passwordForm = document.getElementById('password-form');
var oldPasswordInput = document.getElementById('old-password');
var newPasswordInput = document.getElementById('new-password');
var confirmPasswordInput = document.getElementById('confirm-password');
passwordForm.addEventListener('submit', function(e) {
e.preventDefault();
// 检查旧密码长度
if (oldPasswordInput.value.length < 6 || oldPasswordInput.value.length > 12) {
oldPasswordInput.classList.add('error');
oldPasswordInput.value = '';
oldPasswordInput.placeholder = '旧密码长度有误';
return;
} else {
oldPasswordInput.classList.remove('error');
oldPasswordInput.placeholder = '';
}
// 检查新密码和确认密码是否一致
if (newPasswordInput.value !== confirmPasswordInput.value) {
confirmPasswordInput.classList.add('error');
confirmPasswordInput.value = '';
confirmPasswordInput.placeholder = '新密码与确认密码不一致';
return;
} else {
confirmPasswordInput.classList.remove('error');
confirmPasswordInput.placeholder = '';
}
// 检查新密码是否包含数字、字母和特殊符号
var reg = /^(?=.*[0-9])(?=.*[a-zA-Z])(?=.*[!@#$%^&*]).{6,12}$/;
if (!reg.test(newPasswordInput.value)) {
newPasswordInput.classList.add('error');
newPasswordInput.value = '';
newPasswordInput.placeholder = '必须包含数字、字母和特殊符号';
confirmPasswordInput.value = '';
return;
} else {
newPasswordInput.classList.remove('error');
newPasswordInput.placeholder = '';
}
// 提交表单
passwordForm.submit();
});
</script>
</body>
</html>
```
阅读全文
相关推荐
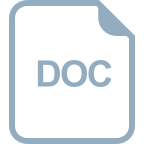
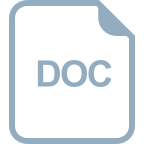
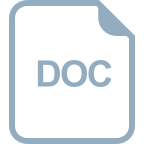
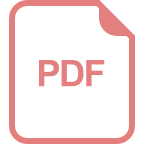
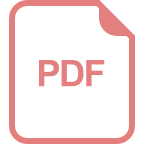
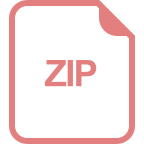
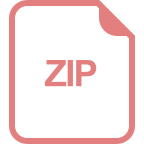
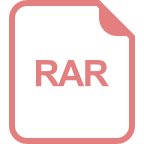
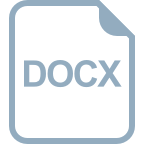
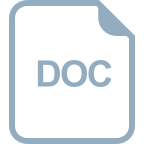
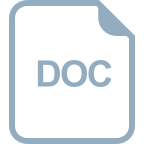
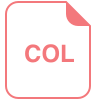
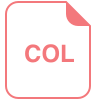





