怎么使用SQLite存储bitmap
时间: 2024-05-02 07:16:09 浏览: 17
SQLite是一种轻型的关系型数据库,不支持直接存储bitmap。但可以将bitmap转换为byte数组后存储在SQLite中。
以下是一个示例代码:
```java
// 将bitmap转换为byte数组
ByteArrayOutputStream stream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.PNG, 100, stream);
byte[] byteArray = stream.toByteArray();
// 将byte数组存储在SQLite中
ContentValues values = new ContentValues();
values.put("image", byteArray);
database.insert(TABLE_NAME, null, values);
```
其中,`bitmap`是要存储的位图,`TABLE_NAME`是SQLite数据库表的名称,`image`是存储byte数组的列名。在插入数据时,将byte数组放入ContentValues对象中,调用`insert()`方法即可存储。
相关问题
android存储照片sqlite
Android中可以将图片数据存储在SQLite数据库中,一般来说,我们需要将图片数据转化为二进制数据,再将其插入到SQLite数据库中。
下面是一个简单的示例代码,演示了如何将图片数据存储在SQLite数据库中:
```java
// 将图片转化为二进制数据
ByteArrayOutputStream stream = new ByteArrayOutputStream();
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.image);
bitmap.compress(Bitmap.CompressFormat.PNG, 100, stream);
byte[] imageData = stream.toByteArray();
// 插入数据到SQLite数据库中
SQLiteDatabase db = dbHelper.getWritableDatabase();
ContentValues values = new ContentValues();
values.put("name", "image1");
values.put("data", imageData);
db.insert("image_table", null, values);
```
在上述代码中,我们首先将图片数据转化为二进制数据,并将其存储在一个字节数组中。然后,我们创建一个ContentValues对象,将图片数据和图片名称插入到其中,并将其插入到SQLite数据库中。
当我们需要读取存储在SQLite数据库中的图片数据时,我们可以使用以下代码:
```java
SQLiteDatabase db = dbHelper.getReadableDatabase();
Cursor cursor = db.rawQuery("SELECT data FROM image_table WHERE name=?", new String[]{"image1"});
if(cursor.moveToFirst()) {
byte[] imageData = cursor.getBlob(0);
Bitmap bitmap = BitmapFactory.decodeByteArray(imageData, 0, imageData.length);
imageView.setImageBitmap(bitmap);
}
```
在上述代码中,我们使用SELECT语句查询了存储在SQLite数据库中的图片数据,并将其转化为Bitmap对象,最后显示在ImageView中。
android sqlite 选择、存储、读取图片完整代码示例
以下是一个完整的Android SQLite选择、存储、读取图片的示例代码:
```java
public class DBHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "images.db";
private static final int DATABASE_VERSION = 1;
public DBHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("CREATE TABLE images (_id INTEGER PRIMARY KEY AUTOINCREMENT, image BLOB)");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS images");
onCreate(db);
}
public void insertImage(Bitmap bitmap) {
byte[] imageBytes = getBytesFromBitmap(bitmap);
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put("image", imageBytes);
db.insert("images", null, values);
db.close();
}
public Bitmap getImage(int id) {
SQLiteDatabase db = this.getReadableDatabase();
String[] columns = new String[] { "image" };
String whereClause = "_id=?";
String[] whereArgs = new String[] { String.valueOf(id) };
Cursor cursor = db.query("images", columns, whereClause, whereArgs, null, null, null);
if (cursor.moveToFirst()) {
byte[] imageBytes = cursor.getBlob(0);
cursor.close();
db.close();
return BitmapFactory.decodeByteArray(imageBytes, 0, imageBytes.length);
}
cursor.close();
db.close();
return null;
}
public List<Integer> getAllIds() {
List<Integer> ids = new ArrayList<>();
SQLiteDatabase db = this.getReadableDatabase();
String[] columns = new String[] { "_id" };
Cursor cursor = db.query("images", columns, null, null, null, null, null);
while (cursor.moveToNext()) {
int id = cursor.getInt(0);
ids.add(id);
}
cursor.close();
db.close();
return ids;
}
private static byte[] getBytesFromBitmap(Bitmap bitmap) {
ByteArrayOutputStream stream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.PNG, 100, stream);
return stream.toByteArray();
}
}
```
使用示例:
```java
// 将图片插入到数据库中
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.image);
DBHelper dbHelper = new DBHelper(this);
dbHelper.insertImage(bitmap);
// 从数据库中读取图片
int id = 1;
Bitmap image = dbHelper.getImage(id);
// 获取所有图片的ID
List<Integer> ids = dbHelper.getAllIds();
```
相关推荐
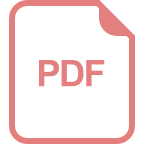
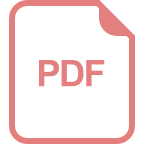
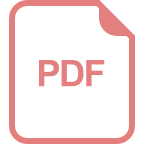












