display_str_heights = [font.getsize(ds)[1] for ds in display_str] AttributeError: 'FreeTypeFont' object has no attribute 'getsize'
时间: 2024-07-28 07:01:24 浏览: 147
看起来你正在尝试使用`getsize`方法从`FreeTypeFont`对象中获取文本的高度,但是遇到了AttributeError。`FreeTypeFont`可能是某些字体处理库(如`PIL`(Python Imaging Library)或`Freetype-Py`)中用于加载自定义字体的对象,它可能不直接支持`getsize`这样的方法。
如果你遇到这个错误,可以尝试以下步骤:
1. **确认库功能**:首先检查你使用的库是否确实提供了`getsize`或类似功能来获取字体尺寸。如果不是,可能需要寻找替代方法,比如使用库提供的其他 API。
2. **文档查找**:查阅库的官方文档,看看是否有其他方法或属性可用于获取文本高度。
3. **使用实例方法**:有时字体对象可能有一个名为`get_size`或`get_pixel_size`的方法,格式可能略有差异,确保你调用的是正确的名称。
4. **自定义计算**:如果上述都不可行,你可能需要编写自己的逻辑来计算文本高度,比如通过遍历每个字符并累加它们的宽度。
由于`getsize`不是标准的字体处理操作,具体解决办法会取决于你在使用的库上下文。如果你能提供更具体的库名或上下文,我可以给出更精确的帮助建议。
相关问题
root.resizable(0, 0) root.wm_attributes("-toolwindow", 1) screenwidth = root.winfo_screenwidth() screenheight = root.winfo_screenheight() widths = 300 heights = 100
这段代码是用来控制窗口的大小和位置的。具体来说:
- `root.resizable(0, 0)` 表示窗口不可调整大小。
- `root.wm_attributes("-toolwindow", 1)` 表示将窗口设置为工具栏窗口,这样在任务栏中不会显示窗口图标。
- `screenwidth = root.winfo_screenwidth()` 和 `screenheight = root.winfo_screenheight()` 分别获取屏幕的宽度和高度。
- `widths = 300` 和 `heights = 100` 分别设置窗口的宽度和高度为 300 像素和 100 像素。
如果你想让窗口在屏幕中央打开,可以使用以下代码:
```
root.geometry('%dx%d+%d+%d' % (widths, heights, (screenwidth - widths) / 2, (screenheight - heights) / 2))
```
它会将窗口的左上角位置设置为屏幕中央。
import numpy as np def replacezeroes(data): min_nonzero = np.min(data[np.nonzero(data)]) data[data == 0] = min_nonzero return data # Change the line below, based on U file # Foundation users set it to 20, ESI users set it to 21 LINE = 20 def read_scalar(filename): # Read file file = open(filename, 'r') lines_1 = file.readlines() file.close() num_cells_internal = int(lines_1[LINE].strip('\n')) lines_1 = lines_1[LINE + 2:LINE + 2 + num_cells_internal] for i in range(len(lines_1)): lines_1[i] = lines_1[i].strip('\n') field = np.asarray(lines_1).astype('double').reshape(num_cells_internal, 1) field = replacezeroes(field) return field def read_vector(filename): # Only x,y components file = open(filename, 'r') lines_1 = file.readlines() file.close() num_cells_internal = int(lines_1[LINE].strip('\n')) lines_1 = lines_1[LINE + 2:LINE + 2 + num_cells_internal] for i in range(len(lines_1)): lines_1[i] = lines_1[i].strip('\n') lines_1[i] = lines_1[i].strip('(') lines_1[i] = lines_1[i].strip(')') lines_1[i] = lines_1[i].split() field = np.asarray(lines_1).astype('double')[:, :2] return field if __name__ == '__main__': print('Velocity reader file') heights = [2.0, 1.5, 0.5, 0.75, 1.75, 1.25] total_dataset = [] # Read Cases for i, h in enumerate(heights, start=1): U = read_vector(f'U_{i}') nut = read_scalar(f'nut_{i}') cx = read_scalar(f'cx_{i}') cy = read_scalar(f'cy_{i}') h = np.ones(shape=(np.shape(U)[0], 1), dtype='double') * h temp_dataset = np.concatenate((U, cx, cy, h, nut), axis=-1) total_dataset.append(temp_dataset) total_dataset = np.reshape(total_dataset, (-1, 6)) print(total_dataset.shape) # Save data np.save('Total_dataset.npy', total_dataset) # Save the statistics of the data means = np.mean(total_dataset, axis=0).reshape(1, np.shape(total_dataset)[1]) stds = np.std(total_dataset, axis=0).reshape(1, np.shape(total_dataset)[1]) # Concatenate op_data = np.concatenate((means, stds), axis=0) np.savetxt('means', op_data, delimiter=' ') # Need to write out in OpenFOAM rectangular matrix format print('Means:') print(means) print('Stds:') print(stds)解析python代码,说明读取的数据文件格式
这段代码是一个用于读取数据文件的 Python 脚本。它包含了两个函数:`read_scalar(filename)` 和 `read_vector(filename)`,并使用了 `replacezeroes(data)` 函数来替换数组中的零值。脚本读取名为 `U_1`, `U_2`, ..., `U_6` 的文件,并根据文件中的数据生成一个包含各个高度的数据集。
函数 `read_scalar(filename)` 用于读取标量数据文件。它打开给定的文件,读取指定行数的数据,并将其存储在一个 NumPy 数组中。函数 `read_vector(filename)` 用于读取包含 x 和 y 分量的矢量数据文件。它也打开给定的文件,读取指定行数的数据,并将其存储在一个 NumPy 数组中。
在脚本的主程序中,一共读取了 6 个名为 `U`, `nut`, `cx`, `cy`, `h` 的文件,并将它们的数据分别存储在 `total_dataset` 列表中。然后,通过使用 NumPy 的函数将列表中的数据合并成一个包含 6 列的数组。最后,将合并后的数据保存为 `Total_dataset.npy` 文件,并计算并保存数据的均值和标准差。
这段代码假设数据文件的格式为文本文件,每行包含一个数据值。
阅读全文
相关推荐
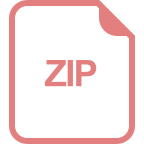
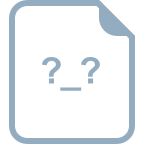












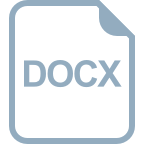
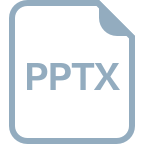