随机森林 累积重要性图
时间: 2024-03-10 08:42:31 浏览: 89
随机森林是一种集成学习方法,它由多个决策树组成。每个决策树都是通过对训练数据进行随机采样和特征随机选择来构建的。随机森林通过对每个决策树的预测结果进行投票或平均来得出最终的预测结果。
累积重要性图是用于评估随机森林中特征的重要性的一种可视化工具。它通过计算每个特征在随机森林中的使用次数和对应的预测准确率之间的关系来衡量特征的重要性。累积重要性图可以帮助我们理解哪些特征对于模型的预测结果起到了关键作用。
相关问题
随机森林相对重要性计算
### 随机森林中特征相对重要性的计算
在随机森林算法中,特征的重要性可以通过多种方式来衡量。一种常用的方法是通过观察某个特征对模型预测性能的影响来进行评估。
#### 基于不纯度的特征重要性
当构建决策树时,每次分裂都会减少节点中的样本集合的不纯度(对于分类问题通常是基尼系数或熵;对于回归问题是方差)。因此可以累积所有基于特定属性的分裂所引起的不纯度减小量作为该属性的重要程度指标之一[^1]。
```python
from sklearn.datasets import load_iris
from sklearn.ensemble import RandomForestClassifier
# 加载数据集
iris = load_iris()
X, y = iris.data, iris.target
# 训练随机森林模型
rf = RandomForestClassifier(n_estimators=100)
rf.fit(X, y)
# 输出各个特征的重要性得分
feature_importances = rf.feature_importances_
print(feature_importances)
```
上述代码展示了如何利用`RandomForestClassifier`类训练一个随机森林分类器,并获取其内部存储的每个输入变量对应的平均降低不纯度值即为这个变量的重要性分数。
#### 排序并展示特征重要性
为了更直观地理解哪些因素最为关键,还可以按照这些数值大小降序排列,并绘制柱状图等形式呈现出来:
```python
import matplotlib.pyplot as plt
import numpy as np
indices = np.argsort(feature_importances)[::-1]
plt.figure(figsize=(8, 6))
plt.title("Feature Importances")
plt.bar(range(len(indices)), feature_importances[indices], align="center", color='r')
plt.xticks(range(len(indices)), indices)
plt.xlim([-1, len(indices)])
plt.show()
```
这段脚本会创建一张图表,其中横轴表示不同维度的位置索引而纵轴则代表相应位置处元素对应原始特征的重要性权重。
#### 使用置换法测量特征重要性
另一种方法是在已经完成建模之后,通过对测试集中某些列的数据进行随机打乱重排操作后再重新评价整体表现变化情况从而间接反映它们各自贡献了多少信息给最终结果解释力上去了多少分量。这种方法被称为“permutation importance”。
```python
from sklearn.inspection import permutation_importance
result = permutation_importance(rf, X, y, n_repeats=10, random_state=42, scoring='accuracy')
sorted_idx = result.importances_mean.argsort()
fig, ax = plt.subplots()
ax.boxplot(result.importances[sorted_idx].T,
vert=False, labels=np.array(iris.feature_names)[sorted_idx])
ax.set_title("Permutation Importance (test set)")
fig.tight_layout()
plt.show()
```
此部分代码实现了对已有的随机森林模型执行多次置换检验过程,并将得到的结果绘制成箱形图以便更好地比较各因子间差异显著与否的程度。
随机森林平均度下降的主要过程
### 随机森林中平均度下降的过程
在随机森林算法中,平均度下降(Mean Decrease Impurity, MDI)用于衡量特征的重要性。MDI 是通过评估某个特征在整个森林中的所有决策树内减少不纯度的程度来实现的。
对于每一个决策树,在每次分割节点时都会计算基尼指数或熵的变化量,这反映了该次分割带来的信息增益。这些变化量会被累积起来并分配给用来做这次分割的那个特征。最终,各个特征的信息增益总和除以总的样本数目就可以得到该特征对整个模型贡献的一个估计值[^1]。
具体来说:
- 对于分类任务,采用的是 Gini 不纯度作为评价标准;
- 而对于回归任务,则可能使用方差或其他类似的指标。
当构建好所有的决策树之后,可以统计每个特征所引起的总体不纯度降低情况,并据此给出各特征重要性的评分。需要注意的是,这种方法可能会偏向那些具有更多类别或者更大数值范围的变量,因为它仅仅考虑了不纯度的绝对减小程度而不涉及其他因素的影响[^4]。
#### 计算过程示例
下面是一个简化版 Python 实现的例子,展示了如何利用 scikit-learn 库获取特征的重要性分数:
```python
from sklearn.datasets import load_iris
from sklearn.ensemble import RandomForestClassifier
# 加载鸢尾花数据集
data = load_iris()
X = data['data']
y = data['target']
# 创建随机森林分类器对象
clf = RandomForestClassifier(n_estimators=100)
# 使用训练数据拟合模型
clf.fit(X, y)
# 输出特征重要性得分
feature_importances = clf.feature_importances_
print(feature_importances)
```
这段代码将会打印出四个特征各自对应的平均度下降值,表示它们分别对目标变量预测能力的影响大小。
阅读全文
相关推荐
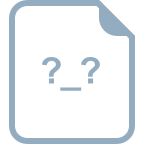















