按照4位二进制加减法计数器的功能表编写VHDL代码
时间: 2024-11-25 12:18:01 浏览: 122
在VHDL中,我们可以创建一个4位二进制加减计数器用于数字系统的设计。这是一个基本的同步计数器,它可以从0开始循环计数到15,然后回到0,可以配置成加法模式或减法模式。以下是简单的VHDL代码示例:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity FourBitBinaryCounter is
Port (
clk : in STD_LOGIC; -- 时钟输入
reset : in STD_LOGIC; -- 外部复位信号
mode : in STD_LOGIC_VECTOR(1 downto 0); -- 计数模式选择(00 - 加法,01 - 减法)
q_out : out STD_LOGIC_VECTOR(3 downto 0) -- 输出四位二进制计数值
);
end FourBitBinaryCounter;
architecture Behavioral of FourBitBinaryCounter is
signal counter_reg : unsigned(3 downto 0); -- 高速内部寄存器
begin
process(clk, reset)
begin
if (reset = '1') then
counter_reg <= (others => '0');
elsif (rising_edge(clk)) then
case mode is
when "00" | "0" => -- 加法模式
counter_reg <= counter_reg + 1;
when "01" | "1" => -- 减法模式
if counter_reg > 0 then
counter_reg <= counter_reg - 1;
else
counter_reg <= (others => '0'); -- 边界条件处理
end if;
when others =>
null; -- 未知模式,通常会触发错误
end case;
end if;
end process;
q_out <= std_logic_vector(counter_reg);
end Behavioral;
-- 相关问题:
-- 1. VHDL中如何实现更复杂的计数器模式?
-- 2. 如何在VHDL中添加中断功能来检测特定计数值?
-- 3. 如果需要扩展到更高位数,应该如何修改此代码结构?
```
这个例子只是一个基础版本,实际应用中可能还需要考虑更多的细节和错误处理。
阅读全文
相关推荐




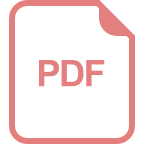

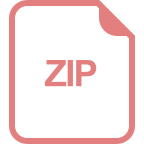







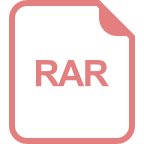
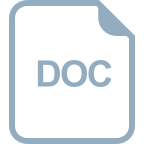
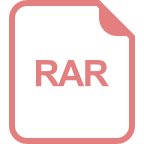

