Match match in Regex.Matches(input, pattern)
时间: 2024-09-24 09:10:25 浏览: 55
在正则表达式(Regex)的`Matches`方法中,"Match Match"通常并不是一个直接的关键字或语法。`Matches`方法是.NET Framework中的一个功能,它用于在一个输入字符串`input`中查找所有匹配给定模式`pattern`的结果。
当你调用`Regex.Matches(input, pattern)`时,它会返回一个`MatchCollection`对象,其中包含了所有找到的匹配项。每个匹配项都是一个`Match`对象,它代表了输入字符串中特定模式的一个实例。你可以通过遍历这个集合来获取每一段匹配的文本、开始和结束位置等信息。
例如:
```csharp
string input = "The quick brown fox jumps over the lazy dog.";
string pattern = @"\b\w+\b"; // 匹配单词
MatchCollection matches = Regex.Matches(input, pattern);
foreach (Match match in matches)
{
Console.WriteLine(match.Value); // 输出匹配到的单词
}
```
在这个例子中,`Match Match`不是作为整体使用的,而是指每次循环迭代中的`Match`对象。
相关问题
c#编程,Regex.Matches("ChC12ChC", @"(Ch[1-6]|Ch[A-F]|LA|LB|VTR1|VTR2|ATR)")怎么去重
在C#编程中,可以使用正则表达式来匹配和提取字符串中的特定模式。在给定的例子中,Regex.Matches方法用于找到字符串中与给定正则表达式匹配的所有子字符串。
要去重这些匹配结果,可以使用HashSet<T>类来存储唯一的匹配项。HashSet<T>是一个集合类,它只包含唯一的元素,并且提供了高效的查找和插入操作。
下面是一个示例代码,演示如何使用HashSet<T>来去重匹配结果:
```csharp
using System;
using System.Collections.Generic;
using System.Text.RegularExpressions;
public class Program
{
public static void Main()
{
string input = "ChC12ChC";
string pattern = @"(Ch[1-6]|Ch[A-F]|LA|LB|VTR1|VTR2|ATR)";
HashSet<string> uniqueMatches = new HashSet<string>();
MatchCollection matches = Regex.Matches(input, pattern);
foreach (Match match in matches)
{
uniqueMatches.Add(match.Value);
}
foreach (string match in uniqueMatches)
{
Console.WriteLine(match);
}
}
}
```
运行上述代码,输出将是去重后的匹配结果:
```
ChC
ChC12
```
vb.net Regex.Match匹配正则表达式中间一个字符
要匹配正则表达式中间的一个字符,可以使用圆括号将该字符括起来,并使用单个点号`.`表示任意字符进行匹配。在VB.NET的Regex.Match方法中,可以使用Groups属性访问匹配到的分组,从而获取中间的字符。
例如,如果要匹配一个由英文单词组成的字符串,并提取每个单词中间的字符,可以使用如下代码:
```vb.net
Dim input As String = "Hello, world! This is a test."
Dim pattern As String = "\b(\w)(\w+)\b"
Dim matches As MatchCollection = Regex.Matches(input, pattern)
For Each match As Match In matches
Dim letter As String = match.Groups(1).Value
Console.WriteLine("Letter in middle: " & letter)
Next
```
在上面的正则表达式中,`\b`表示单词边界,`\w`表示一个字母或数字字符,`(\w)`表示匹配第一个字母,`(\w+)`表示匹配后面的所有字母,最后的`\b`表示单词边界。在循环中,我们可以使用`match.Groups(1).Value`获取到第一个括号中匹配到的字符,即单词中间的字符。
阅读全文
相关推荐



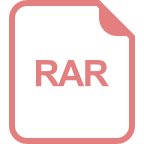











