#include <stdio.h> struct STUDENT { int num; char name[10]; int computer, english, math; /* 三门课程成绩 */ double average; /* 个人平均成绩 */ }; void fun(struct STUDENT s[],struct STUDENT *pmax); /* 平均分最高的学生信息 max , 通过 pmax 改变 */ int main(void) {struct STUDENT max,s[4]={{1,"zhao",88,85,90},{2,"qian",85,85,85},{3,"sun",90,85,90},{4,"li",60,90,90}}; fun(s,&max); /* 平均分最高的学生信息 max , 通过 fun的 pmax 改变 */ printf("num:%d,name:%s,average:%.2lf\n", max.num, max.name, max.average); return 0; } /* 请在这里填写答案 */
时间: 2024-04-12 07:29:08 浏览: 272
你可以在下面填写函数`fun`的具体实现代码:
```c
void fun(struct STUDENT s[], struct STUDENT *pmax) {
double max_average = 0.0;
for (int i = 0; i < 4; i++) {
double average = (s[i].computer + s[i].english + s[i].math) / 3.0;
s[i].average = average; // 计算个人平均成绩
if (average > max_average) {
max_average = average;
*pmax = s[i]; // 更新平均分最高的学生信息
}
}
}
```
这样,调用`fun`函数后,`max`结构体变量中就存储了平均分最高的学生的信息。在`main`函数中打印出学号、姓名和平均分即可。
相关问题
# include <stdio.h> struct student { /* 学生信息结构定义 */ int num; /* 学号 */ char name[10]; /* 姓名 */ int computer, english, math; /* 三门课程成绩 */ double average; /* 个人平均成绩 */ }; int main() { int i, n; struct student stu,max; /* 定义结构变量 */ printf ("Input n: "); scanf ("%d", &n); printf ("Input the student’s number, name and course scores\n"); for (i = 1; i <= n; i++){ printf ("No.%d: ", i); scanf ("%d%s%d%d%d",&stu.num,stu.name,&stu.math,&stu.english,&stu.computer); stu.average = (stu.math + stu.english + stu.computer) / 3.0; if (i == 1){ max = stu; /* 结构变量赋值 */ }else if (max.average < stu.average){ max = stu; } } printf("num:%d, name:%s, average:%.2lf\n", max.num, max.name, max.average); return 0; }向这个代码里面添加一个查询和修改学生的成绩功能
好的,可以尝试如下添加查询和修改学生成绩的代码:
```c++
#include <stdio.h>
#include <string.h>
struct student { /* 学生信息结构定义 */
int num; /* 学号 */
char name[10]; /* 姓名 */
int computer, english, math; /* 三门课程成绩 */
double average; /* 个人平均成绩 */
};
int main() {
int i, n, choice;
struct student stu[100],max; /* 定义结构变量 */
printf ("Input n: ");
scanf ("%d", &n);
printf ("Input the student’s number, name and course scores\n");
for (i = 1; i <= n; i++){
printf ("No.%d: ", i);
scanf ("%d%s%d%d%d",&stu[i].num,stu[i].name,&stu[i].math,&stu[i].english,&stu[i].computer);
stu[i].average = (stu[i].math + stu[i].english + stu[i].computer) / 3.0;
if (i == 1){
max = stu[i]; /* 结构变量赋值 */
}else if (max.average < stu[i].average){
max = stu[i];
}
}
printf("num:%d, name:%s, average:%.2lf\n", max.num, max.name, max.average);
while(1) {
printf("\nWhat do you want to do?\n");
printf("1. Query student's grade\n");
printf("2. Modify student's grade\n");
printf("3. Exit\n");
scanf("%d", &choice);
switch(choice) {
case 1:
int num_query;
printf("Input student's number to query: ");
scanf("%d", &num_query);
for(int j = 1; j <= n; j++) {
if(stu[j].num == num_query) {
printf("Student info: num:%d, name:%s, math:%d, english:%d, computer:%d, average:%.2lf\n", stu[j].num, stu[j].name, stu[j].math, stu[j].english, stu[j].computer, stu[j].average);
break;
}
if(j == n) {
printf("No student found with the number %d\n", num_query);
}
}
break;
case 2:
int num_modify, course_modify, new_grade;
printf("Input student's number to modify: ");
scanf("%d", &num_modify);
printf("Which course grade do you want to modify? (1: math, 2: english, 3: computer) ");
scanf("%d", &course_modify);
printf("Input new grade: ");
scanf("%d", &new_grade);
for(int j = 1; j <= n; j++) {
if(stu[j].num == num_modify) {
switch(course_modify) {
case 1:
stu[j].math = new_grade;
break;
case 2:
stu[j].english = new_grade;
break;
case 3:
stu[j].computer = new_grade;
break;
default:
printf("Invalid input\n");
break;
}
stu[j].average = (stu[j].math + stu[j].english + stu[j].computer) / 3.0;
printf("Grade modified successfully!\n");
printf("New student info: num:%d, name:%s, math:%d, english:%d, computer:%d, average:%.2lf\n", stu[j].num, stu[j].name, stu[j].math, stu[j].english, stu[j].computer, stu[j].average);
break;
}
if(j == n) {
printf("No student found with the number %d\n", num_modify);
}
}
break;
case 3:
printf("Exit program.\n");
return 0;
default:
printf("Invalid input\n");
break;
}
}
return 0;
}
```
这个程序添加了一个 while 循环,让用户可以重复进行查询和修改操作,直到选择退出程序。在查询学生成绩时,用户需要输入学生的学号,程序会遍历学生信息结构体数组,找到对应学号的学生信息并输出。在修改学生成绩时,用户需要输入学生的学号、想要修改的科目编号以及新的成绩,程序会根据这些信息修改对应学生的成绩,并重新计算平均成绩,最后输出修改后的学生信息。
#include<stdio.h> struct student { *学生信息结构定义* int num; 冰学号* char name[10]; 冰姓名* intcomputer,english,math;1*三门课程成繢*/ double average; *个人平均成绩*/ }; int main() int i, n; struct student stu,max; 冰定义结构变量* printf ("Input n: "); scanf ("%d", &n); printf ("Input the student's number, name and course scores\n"); for (i = 1; i <= n; i++){ printf ("No.%d: ", i); scanf %d".&stu.num,stu.name,&stu.math,&stu.english,&stu.computer); stu.average = (stu.math + stu.english + stu.computer) / 3.0; if (i == 1){ max = stu; 冰结构变量赋值*/ Felseif(max.average<stu.average){ max = stu; ["%d%5%d%d 冰结构变量赋值*/ Felseif(max.average<stu.average){ max = stu; ["%d%5%d%d printf("num:%d, max.average); return 0; name:%5, average:%.2Ifn", max.num, max.name, ) return 0; }向这个代码里面添加查询和修改功能
要添加查询和修改功能,需要在主函数中添加相应的代码。下面是修改后的代码:
```c
#include <stdio.h>
#include <string.h>
struct student {
int num; // 学号
char name[10]; // 姓名
int computer; // 计算机成绩
int english; // 英语成绩
int math; // 数学成绩
double average; // 平均成绩
};
int main() {
int i, n, choice, num;
struct student stu, max = {0};
printf("Input n: ");
scanf("%d", &n);
printf("Input the student's number, name and course scores\n");
for (i = 1; i <= n; i++) {
printf("No.%d: ", i);
scanf("%d%s%d%d%d", &stu.num, stu.name, &stu.math, &stu.english, &stu.computer);
stu.average = (stu.math + stu.english + stu.computer) / 3.0;
if (i == 1) {
max = stu;
} else if (max.average < stu.average) {
max = stu;
}
}
printf("num:%d, name:%s, average:%.2f\n", max.num, max.name, max.average);
// 添加查询和修改功能
printf("Enter 1 to query a student's information, 2 to modify a student's information: ");
scanf("%d", &choice);
if (choice == 1) {
printf("Enter the student's number: ");
scanf("%d", &num);
for (i = 1; i <= n; i++) {
printf("No.%d: ", i);
scanf("%d%s%d%d%d", &stu.num, stu.name, &stu.math, &stu.english, &stu.computer);
stu.average = (stu.math + stu.english + stu.computer) / 3.0;
if (stu.num == num) {
printf("num:%d, name:%s, math:%d, english:%d, computer:%d, average:%.2f\n", stu.num, stu.name, stu.math, stu.english, stu.computer, stu.average);
break;
}
}
if (i > n) {
printf("Student not found.\n");
}
} else if (choice == 2) {
printf("Enter the student's number: ");
scanf("%d", &num);
for (i = 1; i <= n; i++) {
printf("No.%d: ", i);
scanf("%d%s%d%d%d", &stu.num, stu.name, &stu.math, &stu.english, &stu.computer);
stu.average = (stu.math + stu.english + stu.computer) / 3.0;
if (stu.num == num) {
printf("Enter the new information:\n");
scanf("%d%s%d%d%d", &stu.num, stu.name, &stu.math, &stu.english, &stu.computer);
stu.average = (stu.math + stu.english + stu.computer) / 3.0;
printf("num:%d, name:%s, math:%d, english:%d, computer:%d, average:%.2f\n", stu.num, stu.name, stu.math, stu.english, stu.computer, stu.average);
break;
}
}
if (i > n) {
printf("Student not found.\n");
}
} else {
printf("Invalid choice.\n");
}
return 0;
}
```
在上面的代码中,我们添加了一个 `choice` 变量,用于选择查询或修改功能。当用户选择查询时,我们需要再次输入要查询的学生的学号,然后遍历学生数组,找到对应学号的学生,输出其信息。当用户选择修改时,我们也需要输入要修改的学生的学号,然后遍历学生数组,找到对应学号的学生,再次输入该学生的信息,更新结构体中的信息。如果找不到对应学号的学生,输出相应的错误信息。
阅读全文
相关推荐










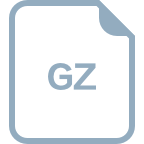
