C语言编写一个程序,可以储存学生的各项基本信息包括学号,姓名,性别,年龄,年级以及三门功课计算机,英语,数学的成绩,然后按照计算机的成绩从高到低进行排序并输出各学生的所以信息
时间: 2023-10-21 10:03:33 浏览: 103
好的,以下是一个基于C语言的程序,可以实现您的需求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENTS 100 // 最多学生数
#define MAX_NAME_LEN 20 // 姓名最大长度
// 学生结构体
struct Student {
int id; // 学号
char name[MAX_NAME_LEN]; // 姓名
char gender; // 性别
int age; // 年龄
int grade; // 年级
int computer_score; // 计算机成绩
int english_score; // 英语成绩
int math_score; // 数学成绩
};
// 学生数组
struct Student students[MAX_STUDENTS];
int num_students = 0; // 当前学生数
// 比较函数,按计算机成绩从高到低排序
int compare(const void *a, const void *b) {
struct Student *s1 = (struct Student *)a;
struct Student *s2 = (struct Student *)b;
return s2->computer_score - s1->computer_score;
}
// 输入一个学生的信息
void input_student() {
struct Student s;
printf("请输入学生信息:\n");
printf("学号:");
scanf("%d", &s.id);
printf("姓名:");
scanf("%s", s.name);
printf("性别:");
scanf(" %c", &s.gender);
printf("年龄:");
scanf("%d", &s.age);
printf("年级:");
scanf("%d", &s.grade);
printf("计算机成绩:");
scanf("%d", &s.computer_score);
printf("英语成绩:");
scanf("%d", &s.english_score);
printf("数学成绩:");
scanf("%d", &s.math_score);
students[num_students++] = s;
}
// 输出所有学生信息
void output_students() {
printf("\n所有学生信息:\n");
for (int i = 0; i < num_students; i++) {
struct Student s = students[i];
printf("学号:%d\t姓名:%s\t性别:%c\t年龄:%d\t年级:%d\t计算机成绩:%d\t英语成绩:%d\t数学成绩:%d\n",
s.id, s.name, s.gender, s.age, s.grade, s.computer_score, s.english_score, s.math_score);
}
}
// 按计算机成绩从高到低排序并输出所有学生信息
void sort_and_output() {
qsort(students, num_students, sizeof(struct Student), compare);
printf("\n按计算机成绩从高到低排序后的学生信息:\n");
for (int i = 0; i < num_students; i++) {
struct Student s = students[i];
printf("学号:%d\t姓名:%s\t性别:%c\t年龄:%d\t年级:%d\t计算机成绩:%d\t英语成绩:%d\t数学成绩:%d\n",
s.id, s.name, s.gender, s.age, s.grade, s.computer_score, s.english_score, s.math_score);
}
}
int main() {
int choice;
do {
printf("\n1. 输入学生信息\n");
printf("2. 输出所有学生信息\n");
printf("3. 按计算机成绩从高到低排序并输出所有学生信息\n");
printf("4. 退出\n");
printf("请选择操作:");
scanf("%d", &choice);
switch (choice) {
case 1:
input_student();
break;
case 2:
output_students();
break;
case 3:
sort_and_output();
break;
case 4:
break;
default:
printf("无效的操作,请重新选择!\n");
break;
}
} while (choice != 4);
return 0;
}
```
程序会让您选择操作,输入1可以输入一个学生的信息,输入2可以输出所有学生信息,输入3可以按计算机成绩从高到低排序并输出所有学生信息,输入4可以退出程序。可以根据需要进行操作。注意,程序中的学生信息是存在内存中的,如果程序结束,信息会丢失。如果需要将信息保存到文件中,可以使用文件读写函数。
阅读全文
相关推荐

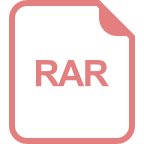












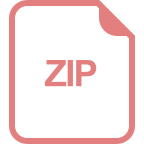