用python进行摄氏度和华氏度之间的转换
时间: 2024-09-25 19:04:44 浏览: 40
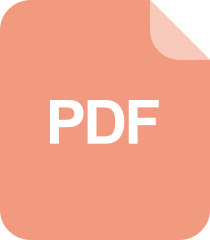
利用Python轻松实现“华氏”和“摄氏”温度的转换
在Python中,我们可以很容易地编写函数来进行摄氏度(℃)和华氏度(℉)之间的转换。以下是两个基本的转换公式:
1. 摄氏度转华氏度:
```python
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 9/5) + 32
return fahrenheit
```
2. 华氏度转摄氏度:
```python
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) * 5/9
return celsius
```
你可以通过调用这两个函数,并传入相应的温度值来进行转换。例如,如果你想将摄氏20度转换为华氏度,可以这样做:
```python
temp_celsius = 20
temp_fahrenheit = celsius_to_fahrenheit(temp_celsius)
print("20°C is", temp_fahrenheit, "°F")
```
同样,如果想把华氏70度转成摄氏,也可以使用`fahrenheit_to_celsius`函数。
阅读全文
相关推荐
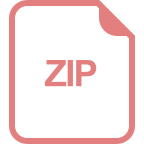















