c语言elf文件段头部表
时间: 2023-10-11 21:03:02 浏览: 140
C语言中的ELF(Executable and Linkable Format)文件段头部表是用来描述ELF文件的各个段的信息的数据结构。ELF文件是一种可执行文件和可链接文件的标准格式,用于在Linux和其他Unix系统上执行和链接程序。
ELF文件段头部表位于ELF文件的头部,用于描述ELF文件的各个段(段是一组相关的数据或代码的有序集合),包括代码段、数据段、BSS段等等。每个段头部表项都包含了段的一些重要信息,如起始地址、大小、访问权限等等。
段头部表的作用是让操作系统或程序加载器能够正确地加载和执行ELF文件的各个段。通过读取段头部表,系统可以确定每个段应该被放置在内存的哪个位置,并且可以根据段的访问权限进行适当的内存保护。此外,段头部表还包含其他一些元数据,如字符串表的偏移量、符号表的偏移量等,这些信息可以帮助调试器和其他工具分析和查找ELF文件的内容。
段头部表是一个固定大小的数据结构,每个表项的大小是固定的,并且表项的数量也是固定的。ELF文件的第一个段头部表项被保留用于描述ELF文件本身,其他表项则用于描述ELF文件中的各个段。程序员可以使用c语言中的结构体来表示段头部表项,并通过读取ELF文件的头部来获取段头部表的起始地址,从而遍历和分析整个段头部表。
总之,C语言中的ELF文件段头部表是用来描述ELF文件的各个段的信息的数据结构,它能够帮助操作系统或程序加载器正确地加载和执行ELF文件,并提供了一些用于分析和查找ELF文件内容的元数据。
相关问题
用C语言写一个ELF文件的解析器
当然可以!以下是一个简单的 ELF 文件解析器的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <elf.h>
int main(int argc, char **argv) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <ELF file>\n", argv[0]);
exit(EXIT_FAILURE);
}
FILE *file = fopen(argv[1], "rb");
if (file == NULL) {
perror("Failed to open file");
exit(EXIT_FAILURE);
}
Elf64_Ehdr header;
if (fread(&header, sizeof(header), 1, file) != 1) {
perror("Failed to read ELF header");
exit(EXIT_FAILURE);
}
printf("ELF header:\n");
printf(" Magic number: %02x %02x %02x %02x\n", header.e_ident[EI_MAG0], header.e_ident[EI_MAG1], header.e_ident[EI_MAG2], header.e_ident[EI_MAG3]);
printf(" Class: %u-bit\n", header.e_ident[EI_CLASS] == ELFCLASS32 ? 32 : 64);
printf(" Data encoding: %s-endian\n", header.e_ident[EI_DATA] == ELFDATA2LSB ? "little" : "big");
printf(" Version: %u\n", header.e_ident[EI_VERSION]);
printf(" OS ABI: %u\n", header.e_ident[EI_OSABI]);
printf(" ABI version: %u\n", header.e_ident[EI_ABIVERSION]);
printf(" Type: %u\n", header.e_type);
printf(" Machine: %u\n", header.e_machine);
printf(" Version: %u\n", header.e_version);
printf(" Entry point address: 0x%lx\n", header.e_entry);
printf(" Program header offset: %lu\n", header.e_phoff);
printf(" Section header offset: %lu\n", header.e_shoff);
printf(" Flags: %u\n", header.e_flags);
printf(" ELF header size: %u\n", header.e_ehsize);
printf(" Program header entry size: %u\n", header.e_phentsize);
printf(" Number of program header entries: %u\n", header.e_phnum);
printf(" Section header entry size: %u\n", header.e_shentsize);
printf(" Number of section header entries: %u\n", header.e_shnum);
printf(" Section name string table index: %u\n", header.e_shstrndx);
if (fseek(file, header.e_shoff, SEEK_SET) != 0) {
perror("Failed to seek to section header table");
exit(EXIT_FAILURE);
}
Elf64_Shdr section_header;
for (int i = 0; i < header.e_shnum; i++) {
if (fread(§ion_header, sizeof(section_header), 1, file) != 1) {
perror("Failed to read section header");
exit(EXIT_FAILURE);
}
printf("Section %d:\n", i);
printf(" Name: %u\n", section_header.sh_name);
printf(" Type: %lu\n", section_header.sh_type);
printf(" Flags: %lu\n", section_header.sh_flags);
printf(" Address: 0x%lx\n", section_header.sh_addr);
printf(" Offset: %lu\n", section_header.sh_offset);
printf(" Size: %lu\n", section_header.sh_size);
printf(" Link: %u\n", section_header.sh_link);
printf(" Info: %u\n", section_header.sh_info);
printf(" Address alignment: %lu\n", section_header.sh_addralign);
printf(" Entry size: %lu\n", section_header.sh_entsize);
}
fclose(file);
return EXIT_SUCCESS;
}
```
这个解析器使用标准 C 库函数和 ELF 头文件,打开指定的 ELF 文件,读取和解析 ELF 文件头部信息和节头表信息,并将它们打印到标准输出中。注意这个解析器仅适用于 64 位 ELF 文件,并且没有处理节的内容。
C语言实现获取elf文件section,并还原生成一个新的link script
可以使用libelf库来获取elf文件section,并使用生成的section信息来编写新的link script。具体步骤包括:
1. 打开指定的elf文件,并获取相关的elf头部信息。
2. 遍历所有的section,找到感兴趣的section,例如.text或.data。
3. 获取每个section的大小、对齐方式、地址等信息。
4. 根据获取到的section信息,生成新的link script,包括定义新的section和设置对齐方式等。
5. 使用生成的新link script进行编译链接,生成新的可执行文件。
需要注意的是,不同的elf文件可能会有不同的section信息,因此具体的实现方式需要根据实际情况进行调整。
阅读全文
相关推荐
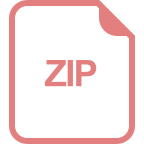
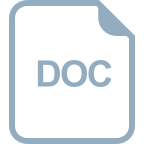
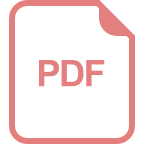
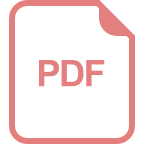
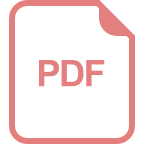
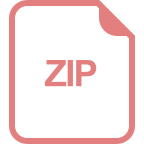
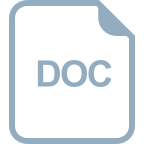
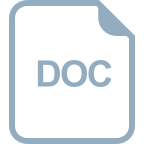
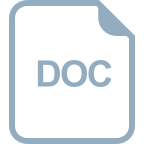
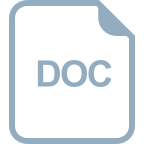
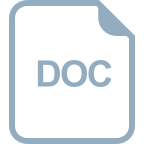
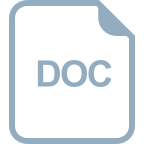
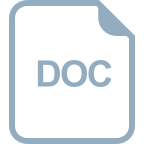
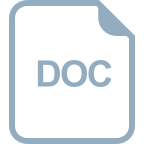
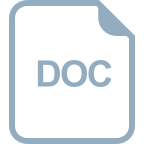
