怎么检查配置是否正确
时间: 2024-09-30 16:05:14 浏览: 14
在C++中,检查配置是否正确通常涉及到对程序初始化时预定义常量、环境变量或配置文件的验证。以下是一些基本步骤:
1. **静态配置**:如果配置信息是在编译时设置的,例如宏定义(`#define`)或编译器选项,你可以使用`#ifdef`或`#ifndef`检查这些定义是否存在。
```cpp
#include <iostream>
// 检查编译时配置
#ifdef ENABLE_FEATURE
std::cout << "Feature is enabled.\n";
#else
std::cerr << "Feature is not enabled. Please recompile with ENABLE_FEATURE defined.\n";
#endif
```
2. **动态配置**:对于运行时可更改的配置,如命令行参数或读取配置文件(如XML、JSON),可以使用标准库(如`<string>`、`<fstream>`)或第三方库(如`configparser`)。
```cpp
#include <iostream>
#include <fstream>
#include <json/json.h> // 假设我们使用了 RapidJSON 库
int main() {
std::string configFile = "config.json";
if (!std::ifstream(configFile)) {
std::cerr << "Failed to open config file.\n";
return 1;
}
Json::Value root;
Json::Reader reader;
bool parsingSuccessful = reader.parseFromFile(root, configFile);
if (!parsingSuccessful) {
std::cerr << "Invalid configuration file format.\n";
return 1;
}
// 检查关键值是否存在
if (root.isMember("feature")) {
std::cout << "Feature is set to: " << root["feature"].asString() << "\n";
} else {
std::cerr << "Required feature not found in the config.\n";
}
}
```
这里的例子假设有一个 JSON 配置文件 `config.json`,包含了一个名为 `"feature"` 的键。你需要根据实际配置结构进行相应的检查。
3. **错误处理**:确保在可能出现错误的地方提供清晰的错误信息,帮助用户识别并修复问题。
**相关问题--:**
1. 如何处理不同类型的配置源(例如环境变量、命令行参数和文件)?
2. C++中如何从命令行获取参数?
3. 如果配置文件不存在或格式不正确,应该如何优雅地处理错误?
阅读全文
相关推荐
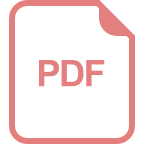
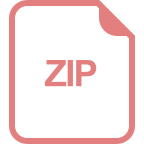
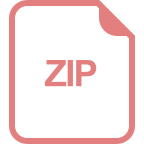
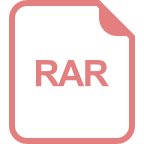
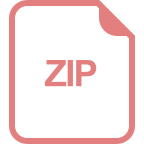
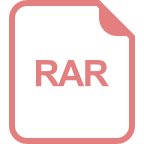
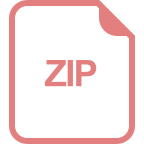
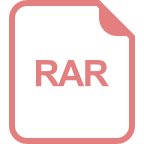
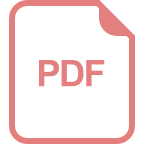
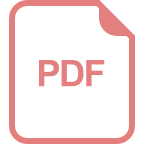
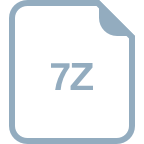
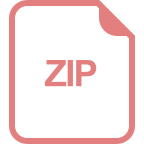
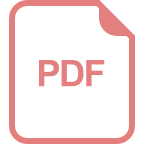
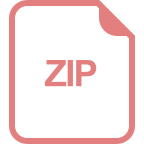
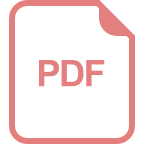
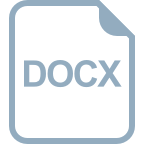
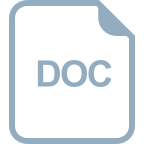