import paramiko import pytest import psutil from common.abs_common import log @pytest.fixture(scope="module") def ssh_client(): print('-----ssh远程连接-------') ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect('10.67.6.56', username='root', password='1') yield ssh ssh.close() print("获取PCIe设备的bus number,带宽和速度") def test_network_interface(ssh_client): stdin, stdout, stderr = ssh_client.exec_command("lspci|grep -i 'non' | cut -f1 -d ' '") interface = stdout.read().decode() stdin, stdout, stderr = ssh_client.exec_command(f"lspci -s {interface} -vvv |grep -i Lnkcap | cut -f2 -d ','") widthcap = stdout.read().decode().strip() outputwidthcap = stdout.read().decode("utf-8") stdin, stdout, stderr = ssh_client.exec_command(f"lspci -s {interface} -vvv |grep -i Lnksta | cut -f2 -d ','") widthsta = stdout.read().decode().strip() outputwidthsta = stdout.read().decode("utf-8") stdin, stdout, stderr = ssh_client.exec_command(f"lspci -s {interface} -vvv |grep -i Lnkcap | cut -f3 -d ','") speedcap = stdout.read().decode().strip() outputspeedcap = stdout.read().decode("utf-8") stdin, stdout, stderr = ssh_client.exec_command(f"lspci -s {interface} -vvv |grep -i Lnksta | cut -f3 -d ','") speedsta = stdout.read().decode().strip() outputspeedsta = stdout.read().decode("utf-8") print("比较lspci中PCIe设备的带宽和速度Lnkcap和Lnksta") assert widthcap == widthsta assert speedcap == speedsta print(outputwidthcap,outputwidthsta,outputspeedcap,outputspeedsta) if __name__ == '__main__': pytest.main(['-o log_cli=True --html=./test_get_nic_width.html -s'])
时间: 2024-04-03 12:32:59 浏览: 21
这段代码是用于测试获取PCIe设备的带宽和速度,其中使用了paramiko库建立远程SSH连接,执行了一些命令来获取相关信息。在test_network_interface函数中,首先使用lspci命令获取PCIe设备的bus number,然后通过grep和cut命令获取Lnkcap和Lnksta信息,分别表示PCIe设备的带宽和速度,最后通过assert语句比较这两个值是否相等。如果测试通过,会输出相关信息,并生成一个HTML格式的测试报告。
相关问题
分析一下这段代码:import paramikossh = paramiko.SSHClient()ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())ssh.connect('192.168.0.1', username='username', password='password')并逐行给出注释与详细解释
好的,以下是这段代码的逐行注释和详细解释:
```
# 导入paramiko库
import paramiko
# 创建SSHClient对象
ssh = paramiko.SSHClient()
# 设置自动添加主机密钥
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
# 连接SSH服务器
ssh.connect('192.168.0.1', username='username', password='password')
```
1. `import paramiko`:导入paramiko库,该库提供了SSH连接的客户端实现,可以通过Python脚本实现自动化连接。
2. `ssh = paramiko.SSHClient()`:创建SSHClient对象,用于与SSH服务器建立连接。
3. `ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())`:设置自动添加主机密钥,可以避免第一次连接时提示是否添加主机密钥的问题。
4. `ssh.connect('192.168.0.1', username='username', password='password')`:连接SSH服务器,指定IP地址、用户名和密码。其中,IP地址为要连接的SSH服务器的地址,用户名和密码为SSH服务器的登录凭证。
需要注意的是,这段代码仅仅是连接SSH服务器的一个示例,实际应用中还需要根据具体情况进行更改。例如,需要根据SSH服务器的操作系统和版本来选择合适的连接方式和命令。同时,还需要考虑SSH连接的安全性和稳定性,以及网络连接的可靠性和速度等因素。
import paramiko def shutdown_remote_system(): # 远程服务器的IP地址和SSH登录信息 remote_host = '192.168.0.106' remote_port = 22 remote_username = 'root' remote_password = '123' # 创建SSH连接 ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect(remote_host, remote_port, remote_username, remote_password) # 执行关机命令 ssh.exec_command('sudo shutdown -h now') # 关闭SSH连接 ssh.close() 缺少是否已关机判断
你的代码是远程执行关机命令的代码,但是确实缺少判断远程系统是否已经关机的部分。
你可以通过在远程服务器上执行一个命令,判断系统是否已经关机。例如,你可以通过 ping 命令测试远程服务器是否可达。
以下是一个示例代码,可以在远程服务器上执行指定的命令并判断系统是否已经关机:
```python
import paramiko
import time
def shutdown_remote_system():
# 远程服务器的IP地址和SSH登录信息
remote_host = '192.168.0.106'
remote_port = 22
remote_username = 'root'
remote_password = '123'
# 创建SSH连接
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(remote_host, remote_port, remote_username, remote_password)
# 执行关机命令,并等待一段时间
ssh.exec_command('sudo shutdown -h now')
time.sleep(10)
# 判断系统是否已经关机
while True:
response = os.system('ping -c 1 ' + remote_host)
if response == 0:
time.sleep(5)
else:
break
# 关闭SSH连接
ssh.close()
```
这段代码中,使用了 `os.system` 函数来执行 ping 命令,判断远程服务器是否可达。如果远程服务器不可达,则说明系统已经关机。循环检测远程服务器是否可达,直到远程服务器不可达时退出循环。
相关推荐
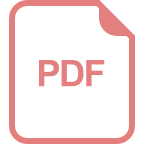
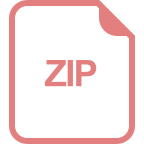
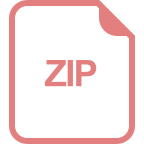












