用C++语言写一个小程序,要求写出完整的代码,该程序使用两个栈,一个栈用来初始化迷宫问题, 另一个用来实现回溯.在用户输入迷宫,一次输入一行.用户输入的迷宫包括任意个行和任意个列.按照用户输入的顺序将行数据放入栈mazeRows中.第二个栈mazeStack在走出迷宫的过程中使用,需要将当前位置为尝试过的临近位置存放到栈中.
时间: 2023-02-07 16:37:23 浏览: 62
以下是使用 C 语言写的一个程序示例,它实现了迷宫问题的初始化和回溯功能,使用了两个栈来实现:
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define ROW_SIZE 100
#define COL_SIZE 100
typedef struct {
int row;
int col;
} Coordinate;
typedef struct {
Coordinate coords[ROW_SIZE * COL_SIZE];
int top;
} Stack;
void stack_init(Stack *stack) {
stack->top = -1;
}
bool stack_is_empty(Stack *stack) {
return stack->top == -1;
}
void stack_push(Stack *stack, Coordinate coord) {
if (stack->top + 1 < ROW_SIZE * COL_SIZE) {
stack->coords[++stack->top] = coord;
}
}
Coordinate stack_pop(Stack *stack) {
if (stack_is_empty(stack)) {
Coordinate coord = {-1, -1};
return coord;
}
return stack->coords[stack->top--];
}
// 下面是程序的主体部分
int main(int argc, char *argv[]) {
char maze[ROW_SIZE][COL_SIZE];
Stack mazeRows, mazeStack;
stack_init(&mazeRows);
stack_init(&mazeStack);
printf("请输入迷宫,一次输入一行:\n");
while (scanf("%s", maze[mazeRows.top + 1]) != EOF) {
stack_push(&mazeRows, (Coordinate){mazeRows.top + 1, 0});
}
Coordinate current = stack_pop(&mazeRows);
while (!stack_is_empty(&mazeRows) || current.row != -1) {
// 在这里处理当前位置
// 如果当前位置是出口,就跳出循环
// 否则,尝试从当前位置向四周走
// 如果可以向某个方向走,就将当前位置压入栈中,并更新当前位置
// 否则,弹出栈顶元素,
阅读全文
相关推荐
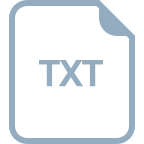

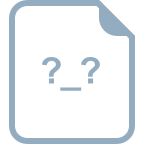
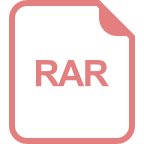
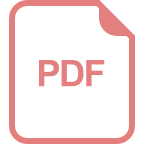
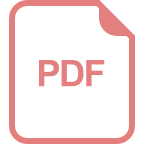
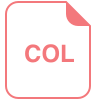
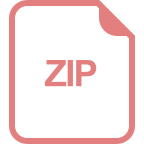