java实现抽签抓阄(附完整源码)
时间: 2023-11-09 12:06:47 浏览: 147
下面是一个简单的Java实现抽签抓阄的代码,包括了两个版本:一个是基于命令行的文本版本,另一个是基于Java Swing的GUI版本。
首先是文本版本的抽签抓阄代码。这个代码实现了一个简单的抽签抓阄程序,用户可以输入参与者的名字,然后程序将随机打乱名字的顺序,并依次输出每个名字,实现抽签抓阄的功能。
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
List<String> names = new ArrayList<>();
// Input the names of participants
System.out.println("Please input the names of the participants (one name per line, press Enter to finish):");
while (true) {
String name = scanner.nextLine().trim();
if (name.isEmpty()) {
break;
}
names.add(name);
}
// Shuffle the order of names
Collections.shuffle(names);
// Output the names in random order
System.out.println("The order of drawing lots is:");
for (String name : names) {
System.out.println(name);
}
}
}
```
接下来是GUI版本的抽签抓阄代码。这个代码使用Java Swing实现了一个简单的用户界面,用户可以在其中输入参与者的名字,点击"Draw Lots"按钮进行抽签抓阄,然后程序将随机打乱名字的顺序,并依次在界面中输出每个名字。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class MainGUI extends JFrame implements ActionListener {
private JTextField nameField;
private JTextArea resultArea;
private List<String> names;
public MainGUI() {
super("Draw Lots");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Initialize the components
JLabel nameLabel = new JLabel("Name:");
nameField = new JTextField(20);
JButton addButton = new JButton("Add");
addButton.addActionListener(this);
JButton drawLotsButton = new JButton("Draw Lots");
drawLotsButton.addActionListener(this);
resultArea = new JTextArea(10, 20);
resultArea.setEditable(false);
// Add the components to the frame
JPanel panel = new JPanel(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
c.gridx = 0;
c.gridy = 0;
c.anchor = GridBagConstraints.LINE_END;
panel.add(nameLabel, c);
c.gridx = 1;
c.anchor = GridBagConstraints.LINE_START;
panel.add(nameField, c);
c.gridx = 2;
panel.add(addButton, c);
c.gridx = 0;
c.gridy = 1;
c.gridwidth = 3;
panel.add(drawLotsButton, c);
c.gridy = 2;
panel.add(new JScrollPane(resultArea), c);
add(panel);
// Initialize the names list
names = new ArrayList<>();
// Show the frame
pack();
setLocationRelativeTo(null);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
if (command.equals("Add")) {
// Add a name to the list
String name = nameField.getText().trim();
if (!name.isEmpty()) {
names.add(name);
}
nameField.setText("");
} else if (command.equals("Draw Lots")) {
// Shuffle the order of names
Collections.shuffle(names);
// Output the names in random order
resultArea.setText("The order of drawing lots is:\n");
for (String name : names) {
resultArea.append(name + "\n");
}
}
}
public static void main(String[] args) {
new MainGUI();
}
}
```
以上代码实现了一个简单的抽签抓阄程序,用户可以根据自己的需要选择文本版本或GUI版本进行使用。
阅读全文
相关推荐
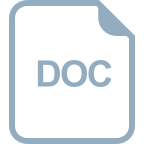
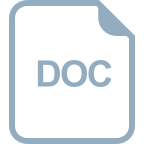
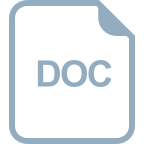
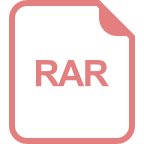
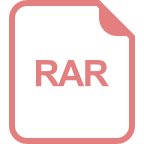
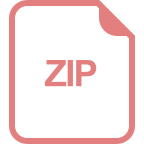
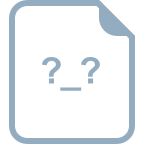
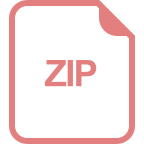
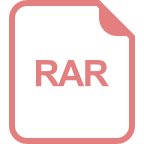
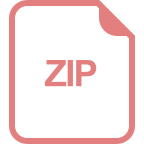
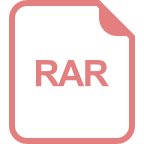
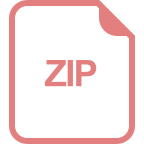
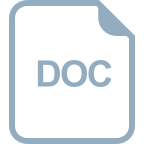
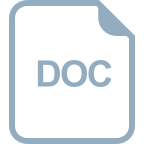
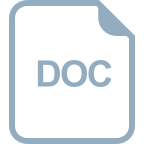

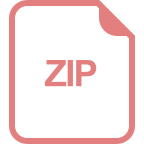