MFC 中DAT文件如何保存Cstring类型
时间: 2024-05-03 15:19:21 浏览: 14
在MFC中,可以使用CStdioFile类来保存Cstring类型的数据到DAT文件中。具体步骤如下:
1. 打开文件
使用CStdioFile类的Open函数打开DAT文件。如果文件不存在,则会自动创建。示例代码如下:
```
CStdioFile file;
if (!file.Open(_T("file.dat"), CFile::modeCreate | CFile::modeWrite))
{
// 文件打开失败
}
```
2. 写入数据
使用CStdioFile类的WriteString函数将Cstring类型的数据写入到DAT文件中。示例代码如下:
```
CString str = _T("Hello, world!");
file.WriteString(str);
```
3. 关闭文件
使用CStdioFile类的Close函数关闭文件。示例代码如下:
```
file.Close();
```
完整代码示例:
```
CStdioFile file;
if (!file.Open(_T("file.dat"), CFile::modeCreate | CFile::modeWrite))
{
// 文件打开失败
return;
}
CString str = _T("Hello, world!");
file.WriteString(str);
file.Close();
```
相关问题
DAT文件如何保存Cstring类型
DAT文件是一种二进制文件格式,它并不直接支持CString类型的保存。不过,我们可以将CString类型的数据转换为字符数组,然后再将字符数组写入到DAT文件中。
下面是一个示例代码:
```c++
#include <fstream>
#include <string>
using namespace std;
int main() {
// 创建一个CString类型的数据
CString str = "Hello, world!";
// 将CString类型的数据转换为字符数组
const char* buf = (const char*)str;
// 打开DAT文件
ofstream fout("data.dat", ios::binary);
// 将字符数组写入到DAT文件中
fout.write(buf, strlen(buf));
// 关闭DAT文件
fout.close();
return 0;
}
```
在上面的示例代码中,我们首先创建了一个CString类型的数据,然后将它转换为字符数组。接着,我们打开一个名为"data.dat"的DAT文件,并将字符数组写入到文件中。最后,我们关闭文件流。
需要注意的是,如果我们要从DAT文件中读取CString类型的数据,那么就需要按照相同的方式将字符数组转换为CString类型。
MFC 如何保存Cstring类型的参数
MFC 中可以使用 CArchive 类来保存 Cstring 类型的参数。CArchive 是一个序列化类,可以将对象以二进制形式进行读写操作。你可以通过在序列化操作中将 Cstring 参数添加到 CArchive 对象中,然后将其保存到文件中。下面是一个简单的示例代码,用于保存 Cstring 参数:
```c++
void SaveString(CString str, CString fileName)
{
CFile file(fileName, CFile::modeCreate | CFile::modeWrite); // 创建文件
CArchive ar(&file, CArchive::store); // 创建序列化对象
ar << str; // 将字符串写入序列化对象
ar.Close(); // 关闭序列化对象
file.Close(); // 关闭文件
}
```
在上述代码中,我们首先创建了一个 CFile 对象,然后使用 CArchive 对象将其打开。接着,我们将 CString 对象添加到序列化对象中,并将其保存到文件中。最后,我们关闭序列化对象和文件。你可以根据自己的需求修改代码,以实现不同的操作。
相关推荐
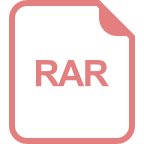












