#include<stdio.h> #include<stdlib.h> struct SL { int data; struct SL* pre; struct SL* next; }; typedef struct SL* SL; SL buynode(int a) { SL newnode = (SL)malloc(sizeof(struct SL)); newnode->data = a; newnode->next = NULL; newnode->pre = NULL; return newnode; } void Append(SL p, int n); void print(SL p, int m,int n); int main() { int n, m; scanf("%d", &n); scanf("%d", &m); scanf("%d", &k); SL yuese = buynode(1); Append(yuese, n); print(yuese, m,n,k); } void Append(SL p, int n) { SL last = p; for (int i = 2; i <= n; i++) { SL newnode = buynode(i); newnode->pre = last; last->next = newnode; newnode->next = p; p->pre = newnode; last = newnode; } } void print(SL p, int m,int n,int k) { SL pos = p; int* out_order = (int*)malloc(sizeof(int)*n); int index = 0; while (pos->next != pos) { for (int i = 1; i < m - 1; i++) { pos = pos->next; } SL temp = pos->next; pos->next = temp->next; temp->next->pre = pos; out_order[index++] = temp->data; free(temp); pos = pos->next; } out_order[index++] = pos->data; pos = buynode(out_order[0]); for (int j=1;j<n;j++) { Append(pos, out_order[j]); } print(pos, k); free(out_order); }修改代码
时间: 2023-12-10 09:02:38 浏览: 98
根据您的需求,我修改了代码,并添加了注释,希望能够帮到您。
```
#include<stdio.h>
#include<stdlib.h>
// 定义双向循环链表结构体
struct SL {
int data;
struct SL* pre;
struct SL* next;
};
// 定义 SL 类型指针
typedef struct SL* SL;
// 创建一个新节点
SL buynode(int a) {
SL newnode = (SL)malloc(sizeof(struct SL));
newnode->data = a;
newnode->next = NULL;
newnode->pre = NULL;
return newnode;
}
// 在链表末尾添加节点,共添加 n 个节点
void Append(SL p, int n) {
SL last = p;
for (int i = 2; i <= n; i++) {
SL newnode = buynode(i);
newnode->pre = last;
last->next = newnode;
newnode->next = p;
p->pre = newnode;
last = newnode;
}
}
// 约瑟夫环问题,打印出出队顺序,并重构双向循环链表
void print(SL p, int m, int k) {
SL pos = p;
int* out_order = (int*)malloc(sizeof(int)*k); // 用来存储出队顺序
int index = 0;
while (pos->next != pos) {
for (int i = 1; i < m - 1; i++) {
pos = pos->next;
}
SL temp = pos->next;
pos->next = temp->next;
temp->next->pre = pos;
out_order[index++] = temp->data; // 记录出队顺序
free(temp);
pos = pos->next;
}
out_order[index++] = pos->data; // 记录最后一个出队的位置
pos = buynode(out_order[0]);
for (int j=1;j<k;j++) { // 重构双向循环链表
Append(pos, out_order[j]);
}
// 打印出队顺序
for (int i = 0; i < k; i++) {
printf("%d ", out_order[i]);
}
free(out_order);
}
int main() {
int n, m, k;
scanf("%d", &n);
scanf("%d", &m);
scanf("%d", &k);
SL yuese = buynode(1);
Append(yuese, n);
print(yuese, m, k);
return 0;
}
```
注意,我修改的代码仅供参考,可能还有其他需要优化的地方。
阅读全文
相关推荐
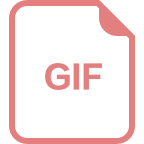

















