下面是函数被调用进行测试的例子。 #include+<stdio.h> #include+<stdlib.h> #define+MAXSIZE+100 #define+LISTINCREMENT+10
时间: 2023-12-24 07:04:25 浏览: 148
下面是函数被调用进行测试的例子:
```
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
#define LISTINCREMENT 10
int main() {
time_t td;
putenv("TZ=PST8PDT");
tzset();
time(&td);
printf("Current time=%s", asctime(localtime(&td)));
struct tm t;
char str[80];
t.tm_sec = 1;
t.tm_min = 3;
t.tm_hour = 7;
t.tm_mday = 22;
t.tm_mon = 11;
t.tm_year = 56;
t.tm_wday = 4;
t.tm_yday = 0;
t.tm_isdst = 0;
strcpy(str, asctime(&t));
printf("%s", str);
return 0;
}
```
相关问题
对以下代码纠正#include<stdio.h> #include<stdlib.h> #include<string.h> #include<math.h> #define MaxSize 100 #define ElemType int #define Status int
这段代码是一个C语言的头文件引用部分,它包含了一些常用的标准库和宏定义。具体解释如下:
1. `#include<stdio.h>`:引用了标准输入输出库,可以使用printf和scanf等函数。
2. `#include<stdlib.h>`:引用了标准库,可以使用malloc和free等函数进行内存管理。
3. `#include<string.h>`:引用了字符串处理库,可以使用strcpy和strlen等函数进行字符串操作。
4. `#include<math.h>`:引用了数学库,可以使用数学函数如sin和cos等。
5. `#define MaxSize 100`:定义了一个宏常量MaxSize,表示数组的最大长度为100。
6. `#define ElemType int`:定义了一个宏常量ElemType,表示数组元素的类型为int。
7. `#define Status int`:定义了一个宏常量Status,表示函数返回的状态类型为int。
这些头文件和宏定义可以提供一些常用的功能和数据类型,方便在代码中使用。如果你有其他问题,请继续提问。
#include <stdio.h> #include <stdlib.h> #include <stdbool.h> #define MaxSize 100 //顺序栈
`#include <stdio.h>`、`#include <stdlib.h>` 和 `#include <stdbool.h>` 这些头文件在C语言程序中分别引入了标准输入输出库(<stdio.h>)、内存管理库(<stdlib.h>)以及布尔类型支持(<stdbool.h>)。`MaxSize 100` 定义了一个常量,表示顺序栈的最大容量为100,通常用于限制栈元素的数量。
顺序栈是一种基于数组实现的数据结构,它按照先进后出(LIFO,Last In First Out)的原则存储和访问数据。在C中,你可以创建一个数组来模拟栈的行为,例如用数组下标作为栈顶指针,当栈满时插入操作会溢出,当栈空时弹出操作会访问到无效位置。
下面是一个简单的顺序栈实现示例:
```c
typedef struct Stack {
int* data; // 存储栈元素的数组
int top; // 栈顶指针
int size; // 栈的实际大小
} Stack;
Stack* createStack() { // 创建栈函数
Stack* stack = (Stack*) malloc(sizeof(Stack));
if (!stack) {
perror("Memory allocation failed");
exit(EXIT_FAILURE);
}
stack->data = (int*) malloc(MaxSize * sizeof(int));
if (!stack->data) {
free(stack); // 如果内存分配失败释放已经分配的部分
perror("Memory allocation failed");
exit(EXIT_FAILURE);
}
stack->top = -1;
stack->size = MaxSize;
return stack;
}
bool push(Stack* stack, int value) { // 入栈操作
if (stack->top == stack->size - 1) {
printf("Stack overflow.\n");
return false;
}
stack->data[++stack->top] = value;
return true;
}
int pop(Stack* stack) { // 出栈操作
if (stack->top == -1) {
printf("Stack underflow.\n");
return -1; // 返回特殊值表示栈空
}
return stack->data[stack->top--];
}
void destroyStack(Stack* stack) { // 销毁栈并释放内存
free(stack->data);
free(stack);
}
```
阅读全文
相关推荐
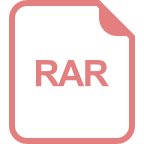














