使用python爬取股票信息
时间: 2023-11-08 09:49:19 浏览: 250
可以使用Python的第三方库requests和BeautifulSoup来爬取股票信息。具体步骤如下:
1. 导入所需库
```python
import requests
from bs4 import BeautifulSoup
```
2. 构造URL
以新浪财经为例,可以根据股票代码构造URL,如下所示:
```python
url = 'http://finance.sina.com.cn/realstock/company/{0}/nc.shtml'.format(stock_code)
```
其中,`stock_code`是需要爬取的股票代码,比如说“600519”代表茅台股票。
3. 发送请求并解析数据
```python
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
```
4. 提取所需数据
根据HTML结构,使用BeautifulSoup提取所需数据,比如说股票名称、当前价格、涨跌幅等信息。
```python
# 获取股票名称
name = soup.find('div', {'class': 'stock-name'}).find('h1').text.strip()
# 获取当前价格
price = soup.find('div', {'class': 'stock-info'}).find('strong').text
# 获取涨跌幅
change = soup.find('div', {'class': 'stock-info'}).find_all('span')[1].text.strip()
```
5. 完整代码示例
```python
import requests
from bs4 import BeautifulSoup
def get_stock_info(stock_code):
url = 'http://finance.sina.com.cn/realstock/company/{0}/nc.shtml'.format(stock_code)
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
name = soup.find('div', {'class': 'stock-name'}).find('h1').text.strip()
price = soup.find('div', {'class': 'stock-info'}).find('strong').text
change = soup.find('div', {'class': 'stock-info'}).find_all('span')[1].text.strip()
print('股票名称:', name)
print('当前价格:', price)
print('涨跌幅:', change)
get_stock_info('600519') # 茅台股票代码
```
输出结果:
```
股票名称: 贵州茅台
当前价格: 1,549.00
涨跌幅: -0.64%
```
阅读全文
相关推荐
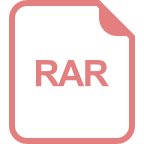
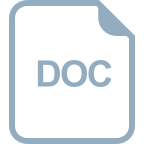
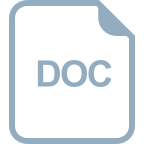

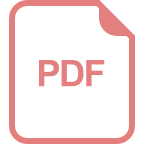





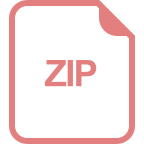
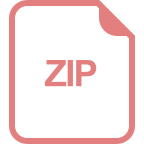