帮我写一个解压zip文件的工具类
时间: 2023-08-28 15:23:17 浏览: 128
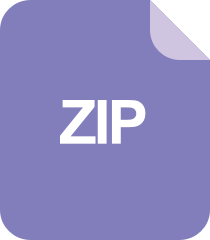
解压zip文件

好的,以下是一个简单的解压 zip 文件的工具类的实现(使用 Java 语言):
```
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Enumeration;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
public class UnzipTool {
public static void unzip(String zipFilePath, String destDir) throws IOException {
File destFile = new File(destDir);
// 如果目标文件夹不存在,则创建
if (!destFile.exists()) {
destFile.mkdir();
}
ZipFile zipFile = new ZipFile(zipFilePath);
// 获取 zip 文件中所有的条目
Enumeration<? extends ZipEntry> entries = zipFile.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
String entryName = entry.getName();
InputStream in = zipFile.getInputStream(entry);
String outPath = destDir + File.separator + entryName;
// 如果是文件夹,则创建文件夹
if (entry.isDirectory()) {
File file = new File(outPath);
file.mkdir();
} else {
// 如果是文件,则写入文件
FileOutputStream out = new FileOutputStream(outPath);
byte[] buffer = new byte[1024];
int len;
while ((len = in.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
in.close();
out.close();
}
}
zipFile.close();
}
}
```
使用方法:
1. 调用 `UnzipTool.unzip(zipFilePath, destDir);` 进行解压,其中 `zipFilePath` 是要解压的 zip 文件路径,`destDir` 是解压后的文件夹路径
2. 解压完成后,zip 文件中的所有文件和文件夹都会被解压到 `destDir` 文件夹中
注意:以上代码只是一个简单的解压 zip 文件的实现,还有很多细节需要考虑,例如文件名编码、文件覆盖、异常处理等。如果需要在实际项目中使用解压工具类,建议使用成熟的第三方库,例如 Apache Commons Compress。
阅读全文
相关推荐
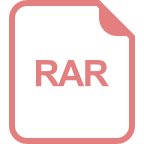
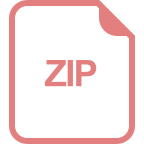
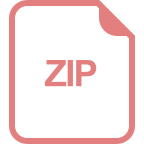
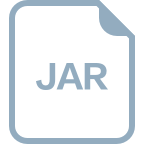
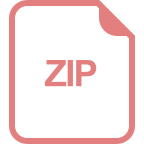
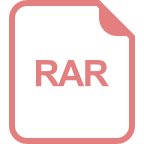
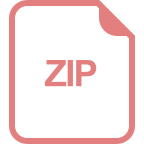
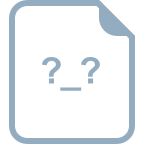
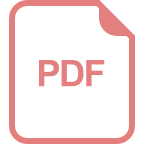
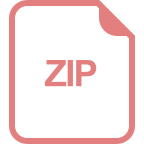
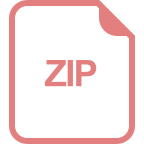
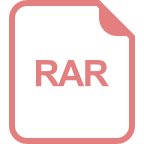