mongoose populate
时间: 2023-10-11 21:04:36 浏览: 59
Mongoose's `populate()` function is used to populate referenced fields in a document with actual data from another collection. It allows you to perform database joins in MongoDB.
Let's say you have two models: `User` and `Post`. The `Post` model has a reference to the `User` model through a field called `author`.
To use `populate()`, you would first define your models using Mongoose:
```javascript
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
name: String,
age: Number,
});
const postSchema = new mongoose.Schema({
title: String,
content: String,
author: {
type: mongoose.Schema.Types.ObjectId,
ref: 'User',
},
});
const User = mongoose.model('User', userSchema);
const Post = mongoose.model('Post', postSchema);
```
Now, let's say you want to find all posts and populate the `author` field with the actual user data. You can do that using the `populate()` function:
```javascript
Post.find().populate('author').exec((err, posts) => {
if (err) {
console.error(err);
return;
}
console.log(posts);
});
```
This will fetch all posts and populate the `author` field with the corresponding user data from the `User` collection. You can then access the populated data using dot notation, like `post.author.name`.
You can also populate multiple fields by passing an array of field names to the `populate()` function, like `populate(['author', 'category'])`.
It's important to note that populate is an expensive operation, as it involves multiple database queries. So, use it judiciously and consider using it only when necessary to avoid performance issues.
相关推荐
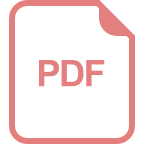
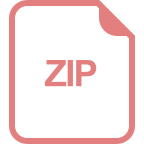
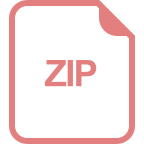














