c++中cin.bad()是什么
时间: 2024-10-07 11:02:45 浏览: 55
在C++中,`cin`是一个输入流对象,它代表程序的标准输入流(通常是从键盘)。当你尝试从`cin`读取数据时,它会进行一系列的验证,例如检查输入是否有效、是否结束等。如果这些验证失败,`cin`会设置一个内部标志,表示当前状态异常,这时你可以使用`cin.bad()`函数来检查这个异常。
当`cin`返回`true`时,意味着输入出现了错误,可能是由于格式错误、EOF(end of file)或其他不可预见的情况。例如,如果你试图读取一个整数,但是用户输入的不是一个有效的整数格式,`cin`就会标记为坏,并调用`bad()`会返回`true`。
```cpp
if (cin.fail()) {
std::cerr << "输入无效!" << std::endl;
cin.clear(); // 清除错误标志
}
if (cin.bad()) {
std::cerr << "输入流损坏!" << std::endl;
// 可能需要进一步处理或关闭输入流
}
```
在处理完错误后,通常会调用`clear()`方法清除错误标志,以便下一次输入操作。
相关问题
cin.clear();不管用
如果你发现`cin.clear()`之后仍然无法正常读取输入,可能是因为还有其他的错误状态关联于`cin`。`cin.clear()`仅仅清除上一次发生的错误,但如果之前发生了多次错误,或者是其他类型的错误(如流结束符),你需要检查并处理所有相关的错误状态。
在C++中,`cin.fail()`、`cin.bad()` 和 `cin.eof()` 分别表示当前流是否有错误、是否损坏以及是否到达流的末尾。你可以结合这些函数一起使用,比如这样:
```cpp
if (cin.fail()) {
std::cout << "输入无效,清除错误并重试:\n";
cin.clear();
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // 忽略错误输入
}
// 然后再尝试获取输入
int value;
if (cin >> value) {
// 成功读取...
} else {
std::cerr << "再次读取失败!\n";
}
```
如果问题依然存在,可能是程序设计的问题或者是输入源的问题,需要深入排查。
#include <iostream>#include <fstream>#include <string>int main() { std::string source_file_name, target_file_name; std::cout << "请输入源文件名:"; std::cin >> source_file_name; std::cout << "请输入目标文件名:"; std::cin >> target_file_name; std::ifstream source_file(source_file_name, std::ios::in | std::ios::binary); std::ofstream target_file(target_file_name, std::ios::out | std::ios::binary); if (!source_file || !target_file) { std::cerr << "文件打开失败" << std::endl; return 1; } target_file << source_file.rdbuf(); if (source_file.bad()) { std::cerr << "读取文件失败" << std::endl; source_file.close(); target_file.close(); return 1; } source_file.close(); target_file.close(); std::cout << "文件复制成功" << std::endl; return 0;}修改这段程序使他运行
这段程序本身是可以运行的,但是需要用户输入源文件名和目标文件名,也就是需要手动输入文件名才能进行复制操作。如果要实现自动化的文件复制,可以将源文件名和目标文件名写入程序中,例如:
```c++
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::string source_file_name = "source.txt"; // 源文件名
std::string target_file_name = "target.txt"; // 目标文件名
std::ifstream source_file(source_file_name, std::ios::in | std::ios::binary);
std::ofstream target_file(target_file_name, std::ios::out | std::ios::binary);
if (!source_file || !target_file) {
std::cerr << "文件打开失败" << std::endl;
return 1;
}
target_file << source_file.rdbuf();
if (source_file.bad()) {
std::cerr << "读取文件失败" << std::endl;
source_file.close();
target_file.close();
return 1;
}
source_file.close();
target_file.close();
std::cout << "文件复制成功" << std::endl;
return 0;
}
```
这样就可以直接运行程序进行文件复制操作,而不需要手动输入文件名。当然,如果需要复制其他文件,只需要修改源文件名和目标文件名即可。
阅读全文
相关推荐
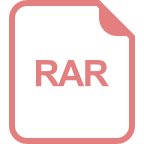
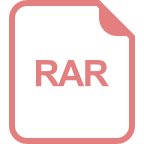
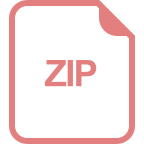
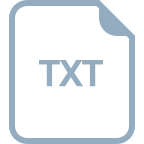
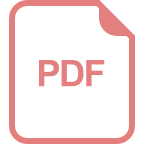
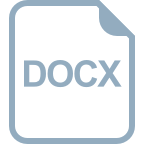
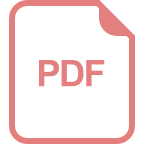
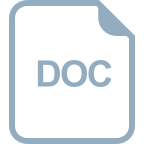
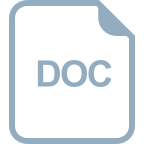
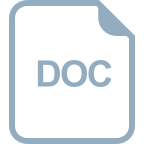
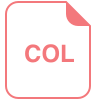




