写一段python代码,使openmv相机能够识别视频左边和右边的图像,若识别到红色或黄色,则发送数据给单片机,并指明在左侧还是右侧
时间: 2023-05-29 16:03:57 浏览: 385
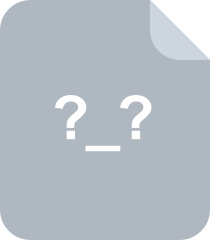
Python-Opencv下摄像头颜色识别

# 导入必要的库
import sensor
import image
import time
import pyb
# 初始化串口通信
uart = pyb.UART(3, 9600, timeout_char=1000)
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
sensor.set_auto_gain(False) # 必须关闭自动增益
sensor.set_auto_whitebal(False) # 必须关闭自动白平衡
# 定义红色和黄色的阈值
red_threshold = (30, 100, 15, 127, 15, 127)
yellow_threshold = (60, 100, -10, 40, -0, 60)
# 定义左侧和右侧的感兴趣区域
left_roi = (0, 0, sensor.width()//2, sensor.height())
right_roi = (sensor.width()//2, 0, sensor.width()//2, sensor.height())
while(True):
# 获取左侧图像
sensor.set_windowing(left_roi)
img_left = sensor.snapshot()
# 获取右侧图像
sensor.set_windowing(right_roi)
img_right = sensor.snapshot()
# 在左侧图像中寻找红色和黄色物体
blobs_left = img_left.find_blobs([red_threshold, yellow_threshold], pixels_threshold=100, area_threshold=100)
if blobs_left:
# 发送数据给单片机,指明在左侧,以及颜色
for blob in blobs_left:
if blob.code() == 1:
uart.write("left,red")
elif blob.code() == 2:
uart.write("left,yellow")
# 在右侧图像中寻找红色和黄色物体
blobs_right = img_right.find_blobs([red_threshold, yellow_threshold], pixels_threshold=100, area_threshold=100)
if blobs_right:
# 发送数据给单片机,指明在右侧,以及颜色
for blob in blobs_right:
if blob.code() == 1:
uart.write("right,red")
elif blob.code() == 2:
uart.write("right,yellow")
阅读全文
相关推荐
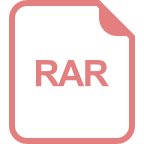
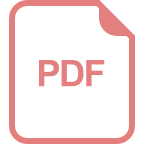
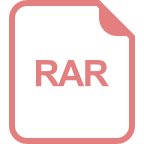
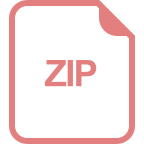
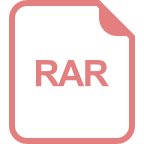
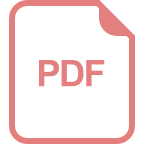
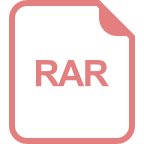
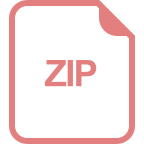
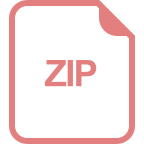
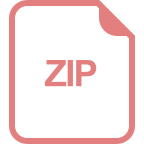
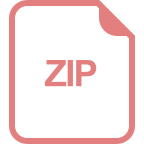
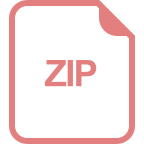
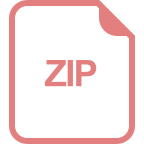
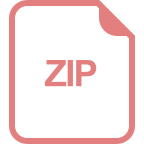