Python编写一个Animal类,包括颜色属性和叫方法,再设计一个Fish类,包括尾巴和颜色两个属性,以及叫方法,fish类继承Animal类,重写构造方法和叫方法
时间: 2024-05-02 19:17:34 浏览: 64
下面是实现代码:
```python
class Animal:
def __init__(self, color):
self.color = color
def make_sound(self):
print("叫")
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
def make_sound(self):
print("吐泡泡")
fish = Fish("红色", "大尾巴")
print(f"{fish.color}的鱼有{fish.tail},会")
fish.make_sound()
```
输出结果:
```
红色的鱼有大尾巴,会
吐泡泡
```
相关问题
用python 设计一个Animal(动物)类,包括颜色属性和叫方法。设计一个Fish(鱼)类,继承自Animal类,包括尾巴和颜色两个属性,以及叫方法,重写构造方法和叫方法。编写代码实现验证。
代码如下:
```python
class Animal:
def __init__(self, color):
self.color = color
def sound(self):
pass
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
def sound(self):
print("鱼不会叫!")
# 实例化一个鱼
fish1 = Fish("红色", "三角尾")
# 输出鱼的颜色和尾巴
print("这条鱼的颜色是{},尾巴是{}".format(fish1.color, fish1.tail))
# 调用鱼的叫方法
fish1.sound()
```
输出结果为:
```
这条鱼的颜色是红色,尾巴是三角尾
鱼不会叫!
```
这段代码定义了一个名为Animal的类,包括颜色属性和叫方法;以及一个继承自Animal的类Fish,包括尾巴和颜色两个属性,以及叫方法。Fish类重写了构造方法和叫方法。然后实例化一个鱼,输出鱼的颜色和尾巴,调用鱼的叫方法。
python创建球类。属性包括球的半径和颜色,计算球体的面积和体积、设计一个Person(人)类,包括姓名,年龄,和血型属性,编写构造方法,用于初始化每个人的具体属性,编写detail方法用于输出每个实例具体值。设计一个Animal(动物)类,包括颜色属性和叫方法,再设计一个Fish类(鱼)类。包括尾巴和颜色两个属性,以及叫法。要求:Fish类继承自Animal类,重写构造方法和叫方法
好的,我可以为您编写这些类的代码。首先是球类的代码:
```python
import math
class Ball:
def __init__(self, radius, color):
self.radius = radius
self.color = color
def area(self):
return 4 * math.pi * self.radius ** 2
def volume(self):
return (4/3) * math.pi * self.radius ** 3
```
接下来是Person类的代码:
```python
class Person:
def __init__(self, name, age, blood_type):
self.name = name
self.age = age
self.blood_type = blood_type
def detail(self):
print("姓名:", self.name)
print("年龄:", self.age)
print("血型:", self.blood_type)
```
然后是Animal和Fish类的代码:
```python
class Animal:
def __init__(self, color):
self.color = color
def sound(self):
print("叫")
class Fish(Animal):
def __init__(self, color, tail, call):
super().__init__(color)
self.tail = tail
self.call = call
def sound(self):
print(self.call)
```
Fish类继承自Animal类,并重写了构造方法和叫方法。
希望这些代码能够帮助到您!
阅读全文
相关推荐
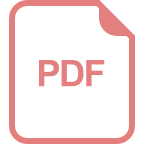
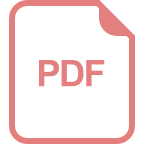




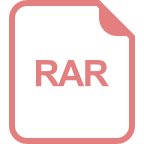
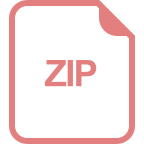
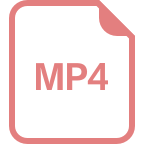
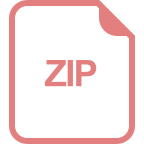
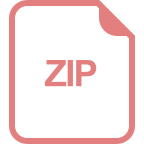