c++中一个string类型的字符串例如“205 只能 5 5”
时间: 2024-02-28 15:57:34 浏览: 17
如果你想将这个字符串进行切分,可以使用stringstream和getline函数来实现。下面是一个示例代码:
```c++
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int main()
{
string str = "205 只能 5 5";
stringstream ss(str);
string temp;
while (getline(ss, temp, ' '))
{
cout << temp << endl;
}
return 0;
}
```
输出结果为:
```
205
只能
5
5
```
这里使用了stringstream将字符串"205 只能 5 5"转化为一个字符流,并使用getline函数按照空格为分隔符进行切分,将切分后的字符串输出到控制台。
相关问题
c++中一个string类型的字符串例如“205 只能 5 5”如何在只能和5之间插入空格
可以使用C++的字符串操作函数来实现这个功能。可以使用`find()`函数查找字符串中"只能"和"5"的位置,然后使用`insert()`函数在这些位置插入空格。代码示例如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str = "205只能55";
int pos1 = str.find("只能"); // 查找"只能"的位置
int pos2 = str.find("5"); // 查找"5"的位置
str.insert(pos1+2, " "); // 在"只能"后面插入空格
str.insert(pos2, " "); // 在"5"前面插入空格
cout << str << endl;
return 0;
}
```
输出结果为:
```
205 只能 5 5
```
这里使用了`find()`函数查找"只能"和"5"的位置,然后使用`insert()`函数在这些位置插入空格。注意插入空格时,需要加上插入位置的偏移量。
c++中一个string类型的字符串例如“205 只能 5 5”如何找第三个和第四个空格的位置,
可以使用循环和`find()`函数来找到第三个和第四个空格的位置。具体来说,可以循环两次,每次使用`find()`函数查找下一个空格的位置,并将查找的起始位置设置为上一个空格的位置加一。代码示例如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str = "205 只能 5 5";
int count = 0; // 记录空格的数量
int pos = -1; // 查找空格的起始位置
while (count < 4)
{
pos = str.find(" ", pos+1); // 从上一个空格的位置加1开始查找下一个空格的位置
if (pos == string::npos) // 如果没找到则退出循环
{
break;
}
count++; // 找到了一个空格,数量加1
}
if (count == 4) // 如果找到了第四个空格
{
cout << "第三个空格的位置:" << str.find(" ", str.find(" ", str.find(" ")+1)+1) << endl;
cout << "第四个空格的位置:" << pos << endl;
}
else
{
cout << "字符串中没有第三个和第四个空格!" << endl;
}
return 0;
}
```
输出结果为:
```
第三个空格的位置:9
第四个空格的位置:12
```
这里使用了循环和`find()`函数来查找第三个和第四个空格的位置。每次查找下一个空格的位置时,需要从上一个空格的位置加一开始查找。如果找到了第四个空格,则输出其位置。注意,如果字符串中没有第三个和第四个空格,则需要特殊处理。
相关推荐
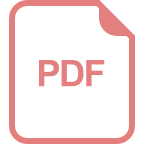
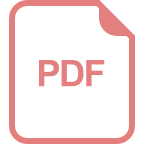
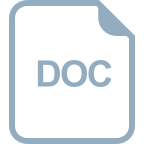









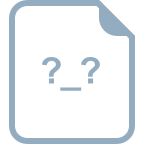
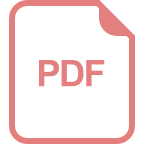
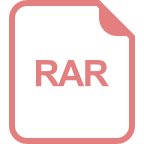
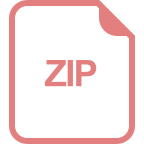