字符串以ascii排序c
时间: 2023-09-19 18:06:19 浏览: 109
要对字符串按照 ASCII 码进行排序,可以使用 Python 中的 `sorted` 函数,其中设置 `key` 参数为 `ord` 函数,表示按照字符的 ASCII 码进行排序。示例如下:
```python
s = "hello world"
sorted_s = ''.join(sorted(s, key=ord))
print(sorted_s) # 输出:dehllloorw
```
在上述示例中,首先定义了一个字符串 `s`,然后使用 `sorted` 函数对其进行排序,排序方法为按照字符的 ASCII 码进行排序,最后使用 `join` 函数将排序后的字符列表合并为一个字符串。
相关问题
字符串的冒泡排序 c语言
字符串的冒泡排序是一种基于冒泡排序算法的字符串排序方法,它可以将一个字符串按照字典序从小到大排序。在C语言中,可以通过比较字符串中每个字符的ASCII码值来实现字符串的比较和排序。具体实现可以参考下面的代码示例:
```c
#include <stdio.h>
#include <string.h>
void bubble_sort(char s[], int k);
int main()
{
char s[100];
int k;
printf("请输入字符串:\n");
scanf("%s", s);
printf("请输入K的值:\n");
scanf("%d", &k);
printf("第%d遍扫描后的中间结果序列为:\n", k);
bubble_sort(s, k);
printf("%s\n", s);
return 0;
}
void bubble_sort(char s[], int k)
{
int i, j, len;
char temp;
len = strlen(s);
for (i = 0; i < k; i++) {
for (j = 0; j < len - i - 1; j++) {
if (s[j] > s[j + 1]) {
temp = s[j];
s[j] = s[j + 1];
s[j + 1] = temp;
}
}
}
}
```
c语言 将字符串列表中的字符串按ascII从小到大排序
可以使用C语言中的qsort函数来进行排序,可以自定义比较函数来指定按照ascII码从小到大排序。示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 自定义比较函数
int cmp(const void *a, const void *b) {
return strcmp(*(char**)a, *(char**)b);
}
int main() {
char *string_list[] = {"apple", "banana", "cat", "dog"};
int n = sizeof(string_list) / sizeof(string_list[0]);
qsort(string_list, n, sizeof(char*), cmp);
for (int i = 0; i < n; i++) {
printf("%s ", string_list[i]);
}
printf("\n");
return 0;
}
```
输出:
```
apple banana cat dog
```
阅读全文
相关推荐
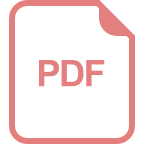

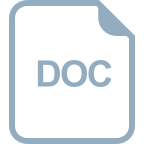











