c语言使用气泡法和选择法将字符串按ASCII码值从小到大排列
时间: 2023-08-10 07:07:32 浏览: 82
好的,以下是使用C语言实现气泡排序和选择排序的代码,可以将字符串按ASCII码值从小到大排列:
使用气泡排序:
```c
#include <stdio.h>
#include <string.h>
void bubble_sort(char str[]) {
int n = strlen(str);
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (str[j] > str[j+1]) {
char temp = str[j];
str[j] = str[j+1];
str[j+1] = temp;
}
}
}
}
int main() {
char str[] = "hello world";
bubble_sort(str);
printf("%s\n", str);
return 0;
}
```
使用选择排序:
```c
#include <stdio.h>
#include <string.h>
void selection_sort(char str[]) {
int n = strlen(str);
for (int i = 0; i < n - 1; i++) {
int min_index = i;
for (int j = i + 1; j < n; j++) {
if (str[j] < str[min_index]) {
min_index = j;
}
}
if (min_index != i) {
char temp = str[i];
str[i] = str[min_index];
str[min_index] = temp;
}
}
}
int main() {
char str[] = "hello world";
selection_sort(str);
printf("%s\n", str);
return 0;
}
```
需要注意的是,在C语言中,字符串其实就是一个字符数组,因此可以直接对字符数组进行排序。同时,也要注意使用`strlen()`函数获取字符串长度,以及使用`char`类型进行字符交换操作。
相关推荐
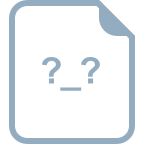














