使用起泡法和选择法将字符串按ASCII码值从小到大排列,C语言
时间: 2024-05-03 21:17:46 浏览: 20
使用起泡法进行排序的C语言代码如下:
```c
#include<stdio.h>
#include<string.h>
void bubbleSort(char arr[], int n){
int i, j;
char temp;
for(i = 0; i < n-1; i++){
for(j = 0; j < n-i-1; j++){
if(arr[j] > arr[j+1]){
temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
int main(){
char str[100];
printf("Enter a string to be sorted: ");
fgets(str, sizeof(str), stdin);
int n = strlen(str);
bubbleSort(str, n-1);
printf("Sorted string: %s", str);
return 0;
}
```
使用选择法进行排序的C语言代码如下:
```c
#include<stdio.h>
#include<string.h>
void selectionSort(char arr[], int n){
int i, j, min_idx;
char temp;
for(i = 0; i < n-1; i++){
min_idx = i;
for(j = i+1; j < n; j++){
if(arr[j] < arr[min_idx]){
min_idx = j;
}
}
temp = arr[min_idx];
arr[min_idx] = arr[i];
arr[i] = temp;
}
}
int main(){
char str[100];
printf("Enter a string to be sorted: ");
fgets(str, sizeof(str), stdin);
int n = strlen(str);
selectionSort(str, n-1);
printf("Sorted string: %s", str);
return 0;
}
```
相关推荐
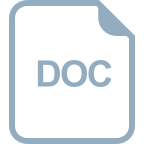
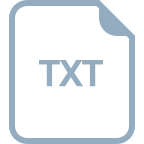
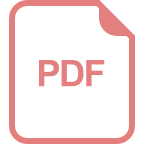














