编写一个C语言程序,用起泡法和选择法将字符串按照ASCII码值从小到大排练
时间: 2024-03-22 13:42:12 浏览: 24
以下是用起泡法将字符串按照ASCII码值从小到大排序的C语言程序:
```c
#include <stdio.h>
#include <string.h>
void bubbleSort(char str[]) {
int n = strlen(str);
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (str[j] > str[j + 1]) {
// 交换相邻两个字符
char temp = str[j];
str[j] = str[j + 1];
str[j + 1] = temp;
}
}
}
}
int main() {
char str[100];
printf("请输入字符串:");
scanf("%s", str);
bubbleSort(str);
printf("排序后的字符串为:%s\n", str);
return 0;
}
```
以下是用选择法将字符串按照ASCII码值从小到大排序的C语言程序:
```c
#include <stdio.h>
#include <string.h>
void selectionSort(char str[]) {
int n = strlen(str);
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (str[j] < str[minIndex]) {
minIndex = j;
}
}
if (minIndex != i) {
// 交换第i个字符和第minIndex个字符
char temp = str[i];
str[i] = str[minIndex];
str[minIndex] = temp;
}
}
}
int main() {
char str[100];
printf("请输入字符串:");
scanf("%s", str);
selectionSort(str);
printf("排序后的字符串为:%s\n", str);
return 0;
}
```
相关推荐
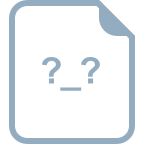














