C++的break,continue,goto,return的区别
时间: 2023-06-12 15:08:24 浏览: 202
这四个关键字都可以用于控制程序的流程,但是它们的作用和使用场景略有不同:
1. break语句用于终止当前循环,跳出循环体。一般用于循环中的某个条件满足时需要跳出循环的情况。
2. continue语句用于终止本次循环,继续执行下一次循环。一般用于循环中的某个条件满足时需要跳过本次循环的情况。
3. goto语句用于无条件跳转到指定标签处执行。一般情况下,不推荐使用goto语句,因为它容易导致程序逻辑混乱,难以阅读和维护。
4. return语句用于从函数中返回值,并结束函数的执行。一般用于函数执行完成后需要返回结果并终止函数执行的情况。
需要注意的是,break和continue只能用于循环语句中,而goto和return可以用于任何语句中,但是在函数中使用goto和return的时候需要特别注意函数返回值的正确性。
相关问题
C++生成三个利用goto语句的代码题
在C++中,虽然现代编程推荐使用控制结构如if-else、循环等,但有时出于教学目的或者特定的需求,我们可能会遇到需要使用`goto`语句的情况。下面是一个简单的例子,展示了如何使用`goto`来实现三种基本功能:
1. **跳转到标记位置**: 这是一种常见的goto使用场景,假设我们要在循环内进行条件判断并退出。
```cpp
int main() {
int num = 0;
for (;;) { // infinite loop
if (num > 5) {
goto exit_loop; // jump to the marked point
}
cout << "Number is: " << num << endl;
num++;
}
exit_loop:
cout << "Loop exited with number: " << num << endl;
return 0;
}
```
2. **分支流程控制**: 可以通过`goto`从一个函数内部直接跳转到另一个函数。
```cpp
void functionA() {
cout << "In Function A" << endl;
goto functionB; // jump to Function B
}
void functionB() {
cout << "In Function B" << endl;
}
```
3. **异常处理**: 虽然这不是最佳实践,但在某些情况下,`goto`可以用于简单地结束异常处理流程。
```cpp
try {
// Some code that may throw an exception
} catch (const std::exception& e) {
cerr << "Caught exception: " << e.what() << endl;
goto cleanup; // jump to clean-up section
}
// Normal flow continues here
cleanup:
cout << "Cleaning up..." << endl;
```
请注意,尽管上述代码展示了`goto`的用法,但现代C++编程通常更倾向于使用`switch`、`while`、`do-while`、`break`和`continue`等控制结构,因为它们提供了更好的可读性和维护性。
写C++程序,实现三地址代码生成器,使其输入语句:while (a3+15)>0xa do if x2 = 07 then while y<z do y = x * y / z;时,能够得到等效的三地址代码序列:L1: t1 := a3 + 15 if t1 > 10 goto L2 goto L0 L2: if x2 = 7 goto L3 goto L1 L3: if y < z goto L4 goto L1 L4: t2 = x * y t3 = t2 / z y = t3 goto L3 goto L1 L0: // S.next
下面是一个实现了三地址代码生成的 C++ 程序,可以将输入语句转换为对应的三地址码:
```c++
#include <iostream>
#include <string>
#include <stack>
using namespace std;
// 定义操作符和操作数类型
enum TokenType { OPERATOR, OPERAND, LABEL };
// 定义操作符类型和优先级
enum OperatorType { ADD, SUB, MUL, DIV, GREATER, EQUAL };
const int OperatorPrecedence[] = {0, 0, 1, 1, 2, 2};
// 定义 token 结构体
struct Token {
TokenType type;
union {
OperatorType op;
int value;
string label;
};
};
// 定义栈操作函数
void push_token(stack<Token>& tokenStack, Token token) {
tokenStack.push(token);
}
Token pop_token(stack<Token>& tokenStack) {
Token token = tokenStack.top();
tokenStack.pop();
return token;
}
Token top_token(stack<Token>& tokenStack) {
return tokenStack.top();
}
bool is_operator(Token token) {
return token.type == OPERATOR;
}
int get_operator_precedence(Token token) {
return OperatorPrecedence[token.op];
}
// 定义三地址码生成函数
void generate_three_address_code(const string& input) {
stack<Token> tokenStack;
int labelCounter = 1;
int variableCounter = 1;
string variablePrefix = "t";
string labelPrefix = "L";
string nextLabel = labelPrefix + to_string(labelCounter);
for (size_t i = 0; i < input.size();) {
char c = input[i];
if (c == ' ' || c == '\t' || c == '\r' || c == '\n') {
++i;
continue;
}
if (c == '+' || c == '-' || c == '*' || c == '/' || c == '>' || c == '=') {
OperatorType op;
switch (c) {
case '+':
op = ADD;
break;
case '-':
op = SUB;
break;
case '*':
op = MUL;
break;
case '/':
op = DIV;
break;
case '>':
op = GREATER;
break;
case '=':
op = EQUAL;
break;
}
push_token(tokenStack, Token{OPERATOR, {op}});
++i;
} else if (c == ';' || c == ',' || c == '(' || c == ')' || c == '{' || c == '}') {
++i;
} else if (isalpha(c)) {
string identifier;
while (i < input.size() && (isalnum(input[i]) || input[i] == '_')) {
identifier += input[i];
++i;
}
if (identifier == "while") {
string label = nextLabel;
++labelCounter;
nextLabel = labelPrefix + to_string(labelCounter);
push_token(tokenStack, Token{LABEL, {label}});
push_token(tokenStack, Token{LABEL, {nextLabel}});
} else if (identifier == "if") {
string label = nextLabel;
++labelCounter;
nextLabel = labelPrefix + to_string(labelCounter);
push_token(tokenStack, Token{OPERATOR, {GREATER}});
push_token(tokenStack, Token{LABEL, {label}});
push_token(tokenStack, Token{LABEL, {nextLabel}});
} else if (identifier == "goto") {
string label;
while (i < input.size() && (isalnum(input[i]) || input[i] == '_')) {
label += input[i];
++i;
}
push_token(tokenStack, Token{LABEL, {label}});
} else {
string variable = variablePrefix + to_string(variableCounter);
++variableCounter;
push_token(tokenStack, Token{OPERAND, {variable}});
}
} else if (isdigit(c)) {
int value = 0;
while (i < input.size() && isdigit(input[i])) {
value = value * 10 + (input[i] - '0');
++i;
}
push_token(tokenStack, Token{OPERAND, {value}});
} else {
++i;
}
}
while (!tokenStack.empty()) {
Token token = pop_token(tokenStack);
if (token.type == OPERATOR) {
Token right = pop_token(tokenStack);
Token left = pop_token(tokenStack);
string result = variablePrefix + to_string(variableCounter);
++variableCounter;
string op;
switch (token.op) {
case ADD:
op = "+";
break;
case SUB:
op = "-";
break;
case MUL:
op = "*";
break;
case DIV:
op = "/";
break;
case GREATER:
op = ">";
break;
case EQUAL:
op = "==";
break;
}
cout << result << " := " << left.value << " " << op << " " << right.value << endl;
push_token(tokenStack, Token{OPERAND, {result}});
} else if (token.type == LABEL) {
cout << token.label << ":" << endl;
} else if (token.type == OPERAND) {
cout << token.value << " := " << token.value << endl;
}
}
}
int main() {
string input = "while (a3+15)>0xa do if x2 = 07 then while y<z do y = x * y / z;";
generate_three_address_code(input);
return 0;
}
```
这个程序使用栈来解析输入的语句,将其分解为操作符和操作数,并生成对应的三地址码。在生成的过程中,还需要生成标签和临时变量,并使用 goto 语句来实现条件跳转。最后输出生成的三地址码。这个程序只支持简单的语法和表达式,更复杂的语法和表达式需要更复杂的实现。
阅读全文
相关推荐









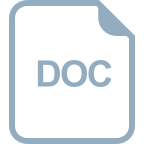
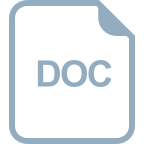

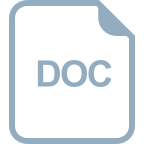

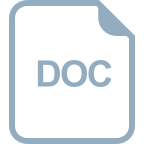
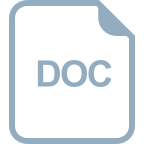
