linux下pthread梯形积分法代码
时间: 2023-08-23 07:05:54 浏览: 56
以下是一个使用 pthread 实现梯形积分法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 4
#define N 1000000
double a = 0.0, b = 1.0;
double h = (b - a) / N;
double integral = 0.0;
void* trapezoidalRule(void* rank) {
long my_rank = (long) rank;
double local_a = a + my_rank * (N / NUM_THREADS) * h;
double local_b = local_a + (N / NUM_THREADS) * h;
double local_integral = 0.0;
for (int i = 1; i <= N / NUM_THREADS; i++) {
double x0 = local_a + (i - 1) * h;
double x1 = local_a + i * h;
local_integral += (x1 - x0) * (sin(x0) + sin(x1)) / 2.0;
}
integral += local_integral;
return NULL;
}
int main(int argc, char* argv[]) {
pthread_t threads[NUM_THREADS];
long thread;
for (thread = 0; thread < NUM_THREADS; thread++) {
pthread_create(&threads[thread], NULL, trapezoidalRule, (void*) thread);
}
for (thread = 0; thread < NUM_THREADS; thread++) {
pthread_join(threads[thread], NULL);
}
printf("With n = %d trapezoids, our estimate\n", N);
printf("of the integral from %f to %f = %.15e\n", a, b, integral);
return 0;
}
```
这个程序将使用 4 个线程并行计算梯形积分,其中 `trapezoidalRule` 函数是每个线程执行的函数。在主函数中,我们创建了 4 个线程,并等待它们完成,并输出最终的积分结果。
相关推荐











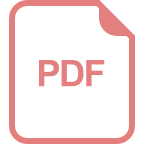
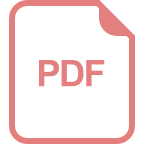