使用Java语言编写一套swing图书管理系统
时间: 2023-07-16 10:13:40 浏览: 99
好的,下面是一个简单的swing图书管理系统的示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.ArrayList;
public class LibrarySystem extends JFrame implements ActionListener {
private JTextField bookTitleField;
private JTextField authorField;
private JTextField isbnField;
private JTextField publisherField;
private JTextField yearField;
private JButton addButton;
private JButton searchButton;
private JButton deleteButton;
private JTextArea resultArea;
private ArrayList<Book> books;
public LibrarySystem() {
super("Library System");
books = new ArrayList<Book>();
JPanel inputPanel = new JPanel(new GridLayout(5, 2));
inputPanel.add(new JLabel("Book Title:"));
bookTitleField = new JTextField();
inputPanel.add(bookTitleField);
inputPanel.add(new JLabel("Author:"));
authorField = new JTextField();
inputPanel.add(authorField);
inputPanel.add(new JLabel("ISBN:"));
isbnField = new JTextField();
inputPanel.add(isbnField);
inputPanel.add(new JLabel("Publisher:"));
publisherField = new JTextField();
inputPanel.add(publisherField);
inputPanel.add(new JLabel("Year:"));
yearField = new JTextField();
inputPanel.add(yearField);
addButton = new JButton("Add");
addButton.addActionListener(this);
searchButton = new JButton("Search");
searchButton.addActionListener(this);
deleteButton = new JButton("Delete");
deleteButton.addActionListener(this);
JPanel buttonPanel = new JPanel(new GridLayout(1, 3));
buttonPanel.add(addButton);
buttonPanel.add(searchButton);
buttonPanel.add(deleteButton);
resultArea = new JTextArea(10, 40);
JScrollPane resultScrollPane = new JScrollPane(resultArea);
setLayout(new BorderLayout());
add(inputPanel, BorderLayout.NORTH);
add(buttonPanel, BorderLayout.CENTER);
add(resultScrollPane, BorderLayout.SOUTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(500, 400);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
String bookTitle = bookTitleField.getText();
String author = authorField.getText();
String isbn = isbnField.getText();
String publisher = publisherField.getText();
String year = yearField.getText();
Book book = new Book(bookTitle, author, isbn, publisher, year);
books.add(book);
resultArea.setText("Book added: " + book);
} else if (e.getSource() == searchButton) {
String searchValue = JOptionPane.showInputDialog(this, "Enter search value:");
StringBuilder sb = new StringBuilder();
for (Book book : books) {
if (book.getTitle().contains(searchValue) || book.getAuthor().contains(searchValue)
|| book.getIsbn().contains(searchValue) || book.getPublisher().contains(searchValue)
|| book.getYear().contains(searchValue)) {
sb.append(book).append("\n");
}
}
resultArea.setText(sb.toString());
} else if (e.getSource() == deleteButton) {
String isbn = JOptionPane.showInputDialog(this, "Enter ISBN of book to delete:");
boolean deleted = false;
for (int i = 0; i < books.size(); i++) {
if (books.get(i).getIsbn().equals(isbn)) {
books.remove(i);
resultArea.setText("Book with ISBN " + isbn + " deleted.");
deleted = true;
break;
}
}
if (!deleted) {
resultArea.setText("Book with ISBN " + isbn + " not found.");
}
}
}
public static void main(String[] args) {
new LibrarySystem();
}
}
class Book {
private String title;
private String author;
private String isbn;
private String publisher;
private String year;
public Book(String title, String author, String isbn, String publisher, String year) {
this.title = title;
this.author = author;
this.isbn = isbn;
this.publisher = publisher;
this.year = year;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getIsbn() {
return isbn;
}
public String getPublisher() {
return publisher;
}
public String getYear() {
return year;
}
public String toString() {
return title + " by " + author + " (" + isbn + "), published by " + publisher + " in " + year;
}
}
```
这个系统可以添加、删除和搜索图书,并且将结果显示在一个文本区域中。你可以根据需要进行修改和扩展。
阅读全文
相关推荐
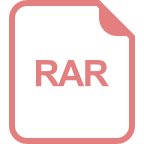
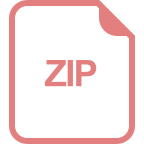
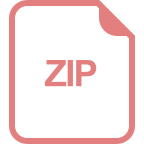
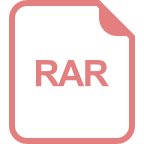
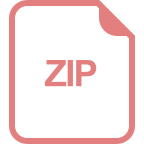

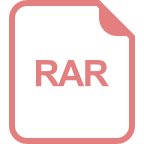
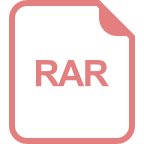
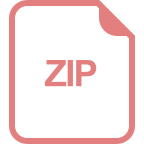
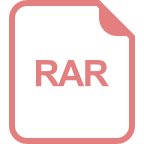
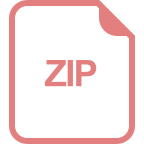
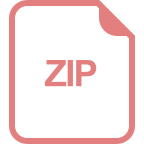
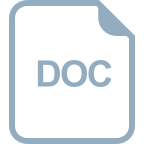
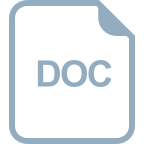
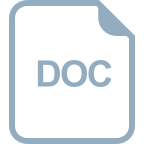
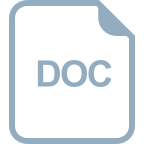
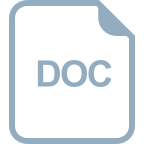
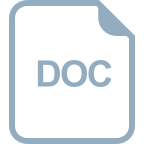
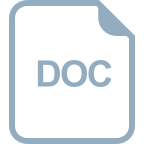