用javaswing写一个图书管理系统
时间: 2023-12-22 12:03:13 浏览: 97
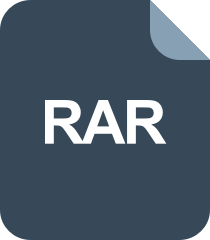
Java Swing编写的图书管理系统

好的,下面是一个简单的Java Swing图书管理系统的例子,你可以参考它来编写自己的系统。这个例子包含了基本的图书管理功能,包括添加、删除、修改和查询图书信息等。
首先,我们需要创建一个Java Swing的GUI界面,用于显示图书管理系统的各个功能模块。下面是一个简单的示例代码:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class BookManager extends JFrame implements ActionListener {
JLabel titleLabel, authorLabel, yearLabel;
JTextField titleText, authorText, yearText;
JButton addButton, deleteButton, updateButton, searchButton;
public BookManager() {
super("图书管理系统");
titleLabel = new JLabel("书名:");
authorLabel = new JLabel("作者:");
yearLabel = new JLabel("年份:");
titleText = new JTextField(20);
authorText = new JTextField(20);
yearText = new JTextField(20);
addButton = new JButton("添加");
deleteButton = new JButton("删除");
updateButton = new JButton("修改");
searchButton = new JButton("查询");
addButton.addActionListener(this);
deleteButton.addActionListener(this);
updateButton.addActionListener(this);
searchButton.addActionListener(this);
JPanel panel = new JPanel(new GridLayout(4, 2));
panel.add(titleLabel);
panel.add(titleText);
panel.add(authorLabel);
panel.add(authorText);
panel.add(yearLabel);
panel.add(yearText);
panel.add(addButton);
panel.add(deleteButton);
panel.add(updateButton);
panel.add(searchButton);
getContentPane().add(panel);
pack();
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
// 添加图书
} else if (e.getSource() == deleteButton) {
// 删除图书
} else if (e.getSource() == updateButton) {
// 修改图书信息
} else if (e.getSource() == searchButton) {
// 查询图书信息
}
}
public static void main(String[] args) {
new BookManager();
}
}
```
在这个例子中,我们创建了一个`JFrame`窗口,包含了4个文本框和4个按钮,用于输入和管理图书信息。我们还实现了`ActionListener`接口,以便响应按钮的点击事件,然后在`actionPerformed`方法中编写相应的图书管理功能。
接下来,我们可以为每个按钮编写具体的图书管理功能代码。例如,当点击“添加”按钮时,我们需要向系统中添加一本新的图书。下面是一个简单的添加图书的代码示例:
```
if (e.getSource() == addButton) {
String title = titleText.getText();
String author = authorText.getText();
String year = yearText.getText();
// 执行添加图书操作
// ...
JOptionPane.showMessageDialog(this, "图书添加成功!");
titleText.setText("");
authorText.setText("");
yearText.setText("");
}
```
在这个代码中,我们从文本框中获取图书的书名、作者和年份信息,然后执行添加图书的操作。最后,我们使用`JOptionPane.showMessageDialog`方法显示一个弹窗,提示用户图书添加成功。
类似地,我们可以编写其他按钮的图书管理功能代码,从而实现一个完整的图书管理系统。
阅读全文
相关推荐
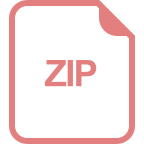
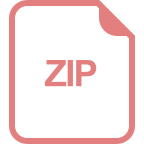
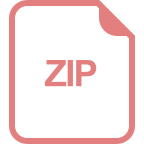
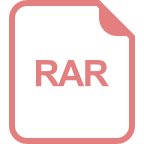
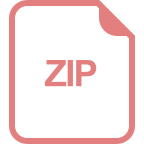
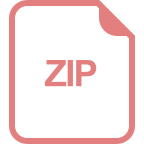
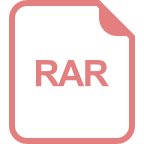
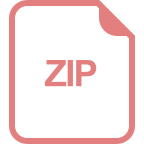
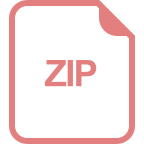
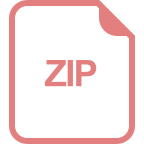
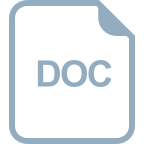
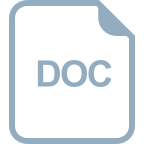
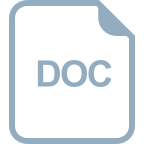
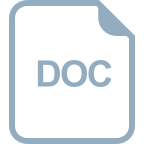



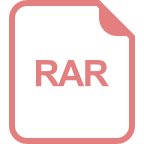