c++实现图书管理系统
时间: 2023-08-30 08:05:09 浏览: 93
下面是一个简单的图书管理系统的 C++ 实现。该系统包含以下功能:
1. 添加图书
2. 删除图书
3. 显示所有图书
4. 搜索图书
5. 借阅图书
6. 归还图书
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 定义图书类
class Book {
public:
Book(string name, string author, int id) : name(name), author(author), id(id), borrowed(false) {}
string getName() const {
return name;
}
string getAuthor() const {
return author;
}
int getId() const {
return id;
}
bool isBorrowed() const {
return borrowed;
}
void setBorrowed(bool borrowed) {
this->borrowed = borrowed;
}
private:
string name;
string author;
int id;
bool borrowed;
};
// 定义图书管理系统类
class Library {
public:
Library() : nextId(1) {}
// 添加图书
void addBook(string name, string author) {
books.push_back(Book(name, author, nextId++));
cout << "添加图书成功!" << endl;
}
// 删除图书
void removeBook(int id) {
auto it = find_if(books.begin(), books.end(), [id](const Book& book) {
return book.getId() == id;
});
if (it != books.end()) {
books.erase(it);
cout << "删除图书成功!" << endl;
} else {
cout << "找不到指定的图书!" << endl;
}
}
// 显示所有图书
void displayAllBooks() {
cout << "所有图书:" << endl;
for (const auto& book : books) {
cout << "ID:" << book.getId() << ",书名:" << book.getName()
<< ",作者:" << book.getAuthor() << ",状态:" << (book.isBorrowed() ? "借出" : "未借出") << endl;
}
}
// 搜索图书
void searchBook(string keyword) {
cout << "搜索结果:" << endl;
for (const auto& book : books) {
if (book.getName().find(keyword) != string::npos || book.getAuthor().find(keyword) != string::npos) {
cout << "ID:" << book.getId() << ",书名:" << book.getName()
<< ",作者:" << book.getAuthor() << ",状态:" << (book.isBorrowed() ? "借出" : "未借出") << endl;
}
}
}
// 借阅图书
void borrowBook(int id) {
auto it = find_if(books.begin(), books.end(), [id](const Book& book) {
return book.getId() == id;
});
if (it != books.end()) {
if (it->isBorrowed()) {
cout << "该图书已被借出!" << endl;
} else {
it->setBorrowed(true);
cout << "借阅成功!" << endl;
}
} else {
cout << "找不到指定的图书!" << endl;
}
}
// 归还图书
void returnBook(int id) {
auto it = find_if(books.begin(), books.end(), [id](const Book& book) {
return book.getId() == id;
});
if (it != books.end()) {
if (it->isBorrowed()) {
it->setBorrowed(false);
cout << "归还成功!" << endl;
} else {
cout << "该图书未被借出!" << endl;
}
} else {
cout << "找不到指定的图书!" << endl;
}
}
private:
vector<Book> books;
int nextId;
};
int main() {
Library library;
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 添加图书" << endl;
cout << "2. 删除图书" << endl;
cout << "3. 显示所有图书" << endl;
cout << "4. 搜索图书" << endl;
cout << "5. 借阅图书" << endl;
cout << "6. 归还图书" << endl;
cout << "0. 退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1: {
cout << "请输入书名和作者:" << endl;
string name, author;
cin >> name >> author;
library.addBook(name, author);
break;
}
case 2: {
cout << "请输入图书 ID:" << endl;
int id;
cin >> id;
library.removeBook(id);
break;
}
case 3: {
library.displayAllBooks();
break;
}
case 4: {
cout << "请输入搜索关键字:" << endl;
string keyword;
cin >> keyword;
library.searchBook(keyword);
break;
}
case 5: {
cout << "请输入图书 ID:" << endl;
int id;
cin >> id;
library.borrowBook(id);
break;
}
case 6: {
cout << "请输入图书 ID:" << endl;
int id;
cin >> id;
library.returnBook(id);
break;
}
case 0: {
return 0;
}
default: {
cout << "无效选择!" << endl;
break;
}
}
}
}
```
这只是一个简单的实现,实际应用中可能需要更复杂的实现,例如数据库存储等。
相关推荐
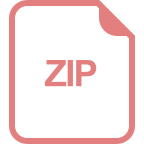
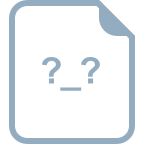














