3.编写程序实现以下功能: (1) 按职工号由小到大的顺序将4个员工的数据(包括号码、姓名、年龄、工资)输出到磁盘文件中保存。 (2) 从键盘输入两个员工的数据(职工号大于已有的职工号),增加到文件的末尾。 (3) 输出文件中全部职工的数据。 (4) 从键盘输入一个号码,在文件中查找有无此职工号,如有则显示此职工是第几个职工,以及此职工的全部数据。如没有,就输出“无此人”。可以反复多次查询,如果输入查找的职工号为0,就结束查询。
时间: 2024-03-08 15:49:16 浏览: 82
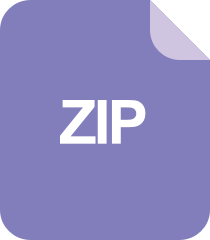
汇编大作业-基于emu8086编写的简易英英词典功能的汇编程序+项目说明.zip

好的,以下是用 Python 编写的程序,实现您所需的功能:
```python
import os
# 定义员工类
class Employee:
def __init__(self, number, name, age, salary):
self.number = number
self.name = name
self.age = age
self.salary = salary
# 重写比较运算符,使得可以按照职工号从小到大排序
def __lt__(self, other):
return self.number < other.number
# 返回员工信息字符串
def __str__(self):
return f"{self.number}\t{self.name}\t{self.age}\t{self.salary}"
# 定义文件名和员工列表
filename = "employees.txt"
employees = []
# 如果文件存在,读取员工数据
if os.path.isfile(filename):
with open(filename, "r") as f:
for line in f:
number, name, age, salary = line.strip().split("\t")
employees.append(Employee(int(number), name, int(age), float(salary)))
# 按职工号从小到大排序
employees.sort()
while True:
# 显示菜单
print("请选择操作:")
print("1. 输出全部职工数据")
print("2. 增加职工数据")
print("3. 查询职工数据")
print("0. 退出程序")
choice = input("请选择操作:")
if choice == "1":
# 输出全部职工数据
print("职工号\t姓名\t年龄\t工资")
for i, employee in enumerate(employees):
print(f"{i+1}\t{employee}")
elif choice == "2":
# 增加职工数据
number = int(input("请输入职工号:"))
name = input("请输入姓名:")
age = int(input("请输入年龄:"))
salary = float(input("请输入工资:"))
employees.append(Employee(number, name, age, salary))
# 按职工号从小到大排序
employees.sort()
with open(filename, "a") as f:
f.write(f"{number}\t{name}\t{age}\t{salary}\n")
elif choice == "3":
# 查询职工数据
number = int(input("请输入要查询的职工号:"))
if number == 0:
break
for i, employee in enumerate(employees):
if employee.number == number:
print(f"职工号为{number}的职工是第{i+1}个职工,其数据为:{employee}")
break
else:
print("无此人")
elif choice == "0":
# 退出程序
break
else:
print("输入有误,请重新输入")
```
注意:该程序默认输入的员工职工号是递增的,如果输入的职工号不是递增的,程序可能会出现错误。
阅读全文
相关推荐
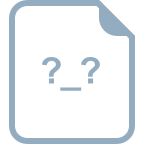
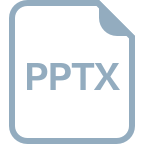













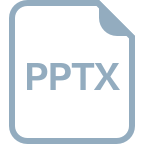
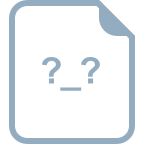
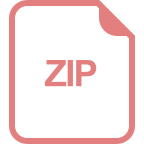