用C++编程实现以下功能: 1 、定义职工类employee:字段成员:职工号num ,姓名name ,年龄age ,工资salary; 成员函数:返回职工号getnum();友元函数:重载运算符>> ,<< 。 2 、编写函数按职工号从小到大的顺序将 5 个职工的数据输出到磁盘文件file1.txt 中保存,int savetofile();该函数返回输入数据的个数。 3 、编写函数从键盘输入一个员工的数据,增加到文件file1.txt 的末尾,int insertfile(int n);插入完成后将新的员工的数量返回。 4 、编写函数从文件中输出全部员工的数据,void outfromfile(int n) 。 5 、编写函数从键盘输入一个号码,从文件中查找有无此职工号,如有则显示此职工 是第几个职工以及此职工的全部数据,如没有,输出“无此人” ,void findfile(int n)。
时间: 2024-03-25 19:38:43 浏览: 271
好的,下面是用 C++ 实现的代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
class employee {
private:
int num, age;
string name;
double salary;
public:
employee(int n = 0, string nm = "", int a = 0, double s = 0.0) : num(n), name(nm), age(a), salary(s) {}
int getnum() const { return num; }
friend ostream& operator<<(ostream& os, const employee& e) {
os << e.num << "\t" << e.name << "\t" << e.age << "\t" << e.salary << endl;
return os;
}
friend istream& operator>>(istream& is, employee& e) {
is >> e.num >> e.name >> e.age >> e.salary;
return is;
}
};
int savetofile(const vector<employee>& employees) {
int count = 0;
ofstream ofs("file1.txt");
if (ofs.is_open()) {
for (const auto& e : employees) {
ofs << e;
count++;
}
ofs.close();
}
return count;
}
int insertfile(int n) {
employee e;
cout << "请输入职工号、姓名、年龄、工资:";
cin >> e;
ofstream ofs("file1.txt", ios::app);
if (ofs.is_open()) {
ofs << e;
ofs.close();
}
return n + 1;
}
void outfromfile(int n) {
ifstream ifs("file1.txt");
if (ifs.is_open()) {
employee e;
while (ifs >> e) {
cout << e;
}
ifs.close();
}
}
void findfile(int n) {
int num, count = 0;
cout << "请输入职工号:";
cin >> num;
ifstream ifs("file1.txt");
if (ifs.is_open()) {
employee e;
while (ifs >> e) {
count++;
if (e.getnum() == num) {
cout << "该职工是第 " << count << " 个职工,职工信息如下:" << endl;
cout << e;
ifs.close();
return;
}
}
ifs.close();
}
cout << "无此人" << endl;
}
int main() {
vector<employee> employees = { {1001, "张三", 25, 5000}, {1002, "李四", 30, 6000},
{1003, "王五", 28, 5500}, {1004, "赵六", 32, 7000},
{1005, "孙七", 27, 5200} };
sort(employees.begin(), employees.end(), [](const employee& e1, const employee& e2) { return e1.getnum() < e2.getnum(); });
int count = savetofile(employees);
cout << "已将 " << count << " 条职工数据保存到文件 file1.txt 中" << endl;
count = insertfile(count);
cout << "已将新职工插入到文件 file1.txt 中,目前职工总数为 " << count << endl;
cout << "从文件 file1.txt 中输出全部职工数据:" << endl;
outfromfile(count);
findfile(count);
return 0;
}
```
在这个程序中,我们定义了一个职工类 `employee`,其中包含了职工号、姓名、年龄和工资等字段成员,以及 `getnum()` 函数和重载的输入输出运算符,用来获取职工号和打印职工信息。接下来,我们实现了以下几个函数:
1. `savetofile()` 函数:用来按职工号从小到大的顺序将 5 个职工的数据输出到磁盘文件 file1.txt 中保存,函数返回输入数据的个数。
2. `insertfile()` 函数:用来从键盘输入一个员工的数据,增加到文件 file1.txt 的末尾,函数返回新的员工的数量。
3. `outfromfile()` 函数:用来从文件中输出全部员工的数据。
4. `findfile()` 函数:用来从键盘输入一个号码,从文件中查找有无此职工号,如有则显示此职工是第几个职工以及此职工的全部数据,如没有,输出“无此人”。
在 `main()` 函数中,我们首先将 5 个职工的数据排序并保存到文件中,然后分别调用上述四个函数来实现各个功能。
阅读全文
相关推荐

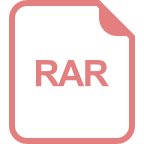















