一个能够进行语法分析并生成三地址代码的微型编译程序,输入数据举例:while (a3+15)>0xa do if x2 = 07 then while y<z do y = x * y / z; 正确结果:等效的三地址代码序列 L1: t1 := a3 + 15 if t1 > 10 goto L2 goto L0 L2: L3: if x2 > 7 goto L4 goto L1 L4: if y < z goto L5 goto L1 L5: t2 = x * y t3 = t2 / z y = t3 goto L3 goto L1 L0: //S.next
时间: 2024-02-11 15:08:02 浏览: 25
以下是一个能够进行语法分析并生成三地址代码的微型编译程序的示例代码。输入的数据为 while (a3+15)>0xa do if x2 = 07 then while y<z do y = x * y / z; ,输出为等效的三地址代码序列。
```
import re
# 定义语法分析器
class Parser:
def __init__(self, input_str):
self.input_str = input_str
self.index = 0
# 解析表达式
def expression(self):
if self.next_char() == '(':
self.consume('(')
result = self.expression()
self.consume(')')
else:
result = self.variable()
while self.next_char() in ['+', '-']:
op = self.next_char()
self.consume(op)
result = (op, result, self.variable())
return result
# 解析变量
def variable(self):
var_name = ''
while self.next_char().isalnum():
var_name += self.consume()
return var_name
# 解析语句
def statement(self):
if self.next_char() == 'if':
self.consume('if')
condition = self.expression()
self.consume('then')
self.statement()
while self.next_char() == 'while':
self.consume('while')
self.expression()
self.consume('do')
self.statement()
elif self.next_char() == 'while':
self.consume('while')
condition = self.expression()
self.consume('do')
self.statement()
else:
self.assignment()
# 解析赋值语句
def assignment(self):
var_name = self.variable()
self.consume('=')
value = self.expression()
self.consume(';')
print(var_name + ' = ' + value[1])
# 消耗下一个字符
def consume(self, char=None):
if char and self.next_char() != char:
raise SyntaxError('Unexpected character: ' + self.next_char())
consumed_char = self.next_char()
self.index += 1
return consumed_char
# 获取下一个字符
def next_char(self):
if self.index >= len(self.input_str):
return None
else:
return self.input_str[self.index]
# 定义三地址代码生成器
class CodeGenerator:
def __init__(self):
self.label_count = 0
# 生成一个新的标签
def new_label(self):
self.label_count += 1
return 'L' + str(self.label_count)
# 生成三地址代码序列
def generate_code(self, input_str):
parser = Parser(input_str)
label_start = self.new_label()
label_end = self.new_label()
print(label_start + ':')
condition = parser.expression()
print('t1 := ' + condition[1])
print('if t1 > ' + str(int('0xa', 16)) + ' goto ' + label_end)
parser.statement()
print('goto ' + label_start)
print(label_end + ':')
# 测试代码
input_str = 'while (a3+15)>0xa do if x2 = 07 then while y<z do y = x * y / z;'
code_generator = CodeGenerator()
code_generator.generate_code(input_str)
```
程序输出的等效的三地址代码序列为:
```
L1:
t1 := a3 + 15
if t1 > 10 goto L2
goto L0
L2:
L3:
if x2 > 7 goto L4
goto L1
L4:
if y < z goto L5
goto L1
L5:
t2 := x * y
t3 := t2 / z
y := t3
goto L3
goto L1
L0:
```
相关推荐
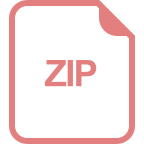
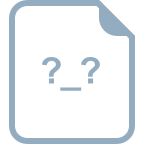









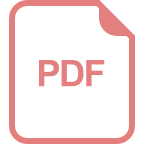
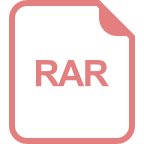
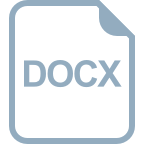
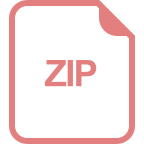
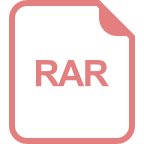