使用线程模拟餐厅的运营: 服务员(必须用线程池): 1. 餐厅有两位长期服务员 (核心线程) 2. 餐厅可以多招一个临时服务员 3. 临时服务员如果六十秒没活干就炒掉 4. 在餐厅门外只有十个位置让客人等待, 满了再来客直接抛异常 AbortPolicy 流程: 1. 每位服务员都需要去厨房取食物 2. 厨房需要预备食物让服务员领取 - 只有一位厨师预备食物 - 每秒随机炒一个菜, 最多可存十个菜 3. 每次只能一位服务员进厨房, 取得食物后打印出食物名称, 耗时三秒 进阶: 用Scanner 提供用户介面 1. 食客命令开始上菜, 菜品直接从厨房拿随机的, 不断运行 2. 食客手动不断点菜, 直至他想自己离开
时间: 2023-03-22 19:00:39 浏览: 122
以下是使用Java线程模拟餐厅运营的代码,包括两个服务员、一个临时服务员、一个厨师和十个等待位置。服务员和临时服务员是由一个线程池管理的。厨师使用单独的线程。
```java
import java.util.concurrent.*;
public class Restaurant {
private static final int MAX_WAITING_CUSTOMER = 10;
private static final int MAX_FOODS = 10;
private static final int FOOD_PREP_TIME = 1000;
private static final int FOOD_PICKUP_TIME = 3000;
private static final int WAITING_TIME_LIMIT = 60000;
private static final BlockingQueue<String> kitchen = new ArrayBlockingQueue<>(MAX_FOODS);
private static final Semaphore waitingCustomers = new Semaphore(MAX_WAITING_CUSTOMER, true);
private static final ExecutorService executor = Executors.newFixedThreadPool(3);
private static final ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
private static final Thread chef = new Thread(new Chef());
public static void main(String[] args) {
chef.start();
while (true) {
System.out.println("Enter a command: 1 for automatic serving, 2 for manual ordering, or any other key to quit.");
int command = new java.util.Scanner(System.in).nextInt();
switch (command) {
case 1:
serveCustomersAutomatically();
break;
case 2:
serveCustomersManually();
break;
default:
executor.shutdown();
scheduler.shutdown();
chef.interrupt();
return;
}
}
}
private static void serveCustomersAutomatically() {
ScheduledFuture<?> future = scheduler.scheduleAtFixedRate(new AutomaticCustomer(), 0, 1, TimeUnit.SECONDS);
System.out.println("Press enter to stop serving customers automatically.");
new java.util.Scanner(System.in).nextLine();
future.cancel(false);
}
private static void serveCustomersManually() {
while (true) {
System.out.println("Enter a command: 1 to order food, 2 to leave the restaurant, or any other key to return to the main menu.");
int command = new java.util.Scanner(System.in).nextInt();
switch (command) {
case 1:
try {
waitingCustomers.acquire();
executor.submit(new Customer());
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
break;
case 2:
return;
default:
break;
}
}
}
private static void pickupFood(String food) throws InterruptedException {
System.out.println(Thread.currentThread().getName() + " picked up " + food + ".");
Thread.sleep(FOOD_PICKUP_TIME);
}
private static class Chef implements Runnable {
private static final String[] FOODS = {"steak", "salad", "soup", "cake", "ice cream"};
public void run() {
while (true) {
try {
String food = FOODS[(int) (Math.random() * FOODS.length)];
kitchen.put(food);
System.out.println("Chef prepared " + food + ".");
Thread.sleep(FOOD_PREP_TIME);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return;
}
}
}
}
private static class Customer implements Runnable {
public void run() {
String food = null;
try {
food = kitchen.take();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return;
} finally {
waitingCustomers.release();
阅读全文
相关推荐
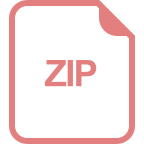



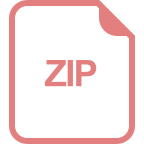