AD9361数据接口FPGA程序
时间: 2024-04-27 19:01:45 浏览: 172
下面是一个简单的Verilog示例,展示了如何在FPGA上实现与AD9361的数据接口通信。这个例子使用了AXI接口和SPI通信。
```verilog
module AD9361_Interface (
input wire clk,
input wire rst,
input wire [15:0] spi_data_in,
output wire [15:0] spi_data_out,
output wire spi_sclk,
output wire spi_cs,
inout wire spi_sdo,
inout wire spi_sdi
);
// AXI slave interface for data read/write
reg [7:0] axi_slave_addr;
reg [31:0] axi_slave_data_in;
wire [31:0] axi_slave_data_out;
wire axi_slave_rd;
wire axi_slave_wr;
// SPI controller
reg spi_enable;
reg [3:0] spi_bit_counter;
// Register map for AD9361
localparam REG_CTRL = 8'h00;
localparam REG_CONFIG = 8'h01;
// Add more register addresses as needed
// Initialize signals
assign spi_data_out = axi_slave_data_out[15:0];
assign axi_slave_data_out[31:16] = 16'b0;
assign spi_sclk = 1'b0;
assign spi_cs = 1'b1;
// SPI enable signal generation (you need to add your own logic to enable/disable SPI controller)
always @(posedge clk or posedge rst) begin
if (rst) begin
spi_enable <= 1'b0;
end else begin
// Add your own logic here to enable/disable SPI controller
// This could be based on a button press, a control signal, or any other condition
spi_enable <= 1'b1;
end
end
// SPI controller
always @(posedge clk or posedge rst) begin
if (rst) begin
spi_bit_counter <= 4'b0;
end else begin
if (spi_enable) begin
spi_bit_counter <= spi_bit_counter + 1;
if (spi_bit_counter == 4'b0) begin
spi_sdo <= 1'b0; // Initialize SDI low
spi_cs <= 1'b0; // Assert CS
spi_sclk <= 1'b1; // Start clock high
end else if (spi_bit_counter == 4'b1) begin
spi_sdo <= axi_slave_rd ? 1'b0 : spi_data_in[15]; // Send MSB of data
spi_sclk <= 1'b0; // Clock low
end else if (spi_bit_counter >= 4'b2 && spi_bit_counter <= 4'b17) begin
spi_sdo <= axi_slave_rd ? 1'b0 : spi_data_in[15 - (spi_bit_counter - 2)]; // Send next bit of data
spi_sclk <= ~spi_sclk; // Toggle clock
end else if (spi_bit_counter == 4'b18) begin
spi_sdo <= axi_slave_rd ? 1'b0 : 1'bz; // Send dummy bit or tri-state SDI
spi_sclk <= ~spi_sclk; // Toggle clock
end else if (spi_bit_counter == 4'b19) begin
spi_sdi <= 1'bz; // Release SDO (tri-state)
spi_sclk <= 1'b1; // Stop clock high
end else if (spi_bit_counter >= 4'b20 && spi_bit_counter <= 4'b35) begin
spi_data_out[15 - (spi_bit_counter - 20)] <= spi_sdi; // Receive next bit of data
spi_sclk <= ~spi_sclk; // Toggle clock
end else if (spi_bit_counter == 4'b36) begin
spi_data_out[15] <= spi_sdi; // Receive LSB of data
spi_cs <= 1'b1; // Deassert CS
spi_enable <= 1'b0; // Disable SPI controller
spi_sclk <= 1'b1; // Stop clock high
end
end else begin
spi_sdo <= 1'bz; // Tri-state SDI
spi_sdi <= 1'bz; // Tri-state SDO
spi_cs <= 1'b1; // Deassert CS
spi_sclk <= 1'b1; // Stop clock high
end
end
end
// AXI slave interface for data read/write
always @(posedge clk or posedge rst) begin
if (rst) begin
axi_slave_addr <= 8'h0;
axi_slave_data_in <= 32'b0;
end else begin
if (axi_slave_wr) begin
case(axi_slave_addr)
REG_CTRL: begin
// Logic to handle write to control register (e.g., enable/disable AD9361)
// You can use axi_slave_data_in to read the control data from AXI bus
// and perform necessary actions to control AD9361.
end
REG_CONFIG: begin
// Logic to handle write to configuration register (e.g., set AD9361 configuration)
// You can use axi_slave_data_in to read the configuration data from AXI bus
// and perform necessary actions to configure AD9361.
end
// Add more cases for other register addresses
default: begin
// Handle write to an unsupported register address
end
endcase
end else if (axi_slave_rd) begin
case(axi_slave_addr)
REG_CTRL: begin
// Logic to handle read from control register (e.g., read status of AD9361)
// You can update axi_slave_data_out to provide the status data to AXI bus.
end
REG_CONFIG: begin
// Logic to handle read from configuration register (e.g., read AD9361 configuration)
// You can update axi_slave_data_out to provide the configuration data to AXI bus.
end
// Add more cases for other register addresses
default: begin
// Handle read from an unsupported register address
axi_slave_data_out <= 32'h0; // Provide default value for unsupported address
end
endcase
end
end
end
endmodule
```
上述代码是一个简单的AD9361数据接口FPGA程序示例,使用AXI接口和SPI通信进行数据读写。请根据实际需求和使用的FPGA平台,对代码进行适当的修改和调整。
需要注意的是,这只是一个基本的框架,您需要根据AD9361的寄存器映射和配置需求,实现适当的逻辑来读写寄存器以及与AD9361进行通信。另外,还需要根据您所使用的FPGA平台和工具链,配置引脚映射和约束文件,以确保正确的信号连接和时序约束。
建议参考ADI提供的参考设计、文档和例程,以更好地了解AD9361的数据接口和FPGA实现。
阅读全文
相关推荐





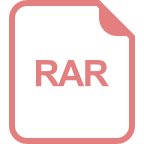


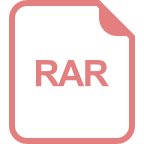







