python将时间转为时辰
时间: 2023-09-12 20:04:48 浏览: 233
### 回答1:
可以使用datetime模块中的strftime函数将时间转为时辰,示例代码如下:
```python
import datetime
now = datetime.datetime.now()
hour = now.strftime("%H")
minute = now.strftime("%M")
print("当前时间为:{}时{}分".format(hour, minute))
```
运行结果为:
```
当前时间为:16时21分
```
### 回答2:
在Python中,可以使用datetime模块来将时间转换为时辰。
首先,我们需要导入datetime模块:
import datetime
然后,我们可以使用datetime模块中的datetime对象来表示时间。假设我们有一个时间对象t,可以通过调用datetime对象的now()方法来获得当前时间:
t = datetime.datetime.now()
我们可以使用strftime()方法将时间对象格式化为指定的字符串形式。时辰可以通过将时间格式化为"%H:%M"的形式来表示。具体的时辰可以通过判断小时数来确定。
例如,我们可以使用以下代码将当前时间转换为时辰:
t = datetime.datetime.now()
time_str = t.strftime("%H:%M")
hour = int(time_str[:2])
if hour < 1:
shichen = "子时"
elif hour < 3:
shichen = "丑时"
elif hour < 5:
shichen = "寅时"
elif hour < 7:
shichen = "卯时"
elif hour < 9:
shichen = "辰时"
elif hour < 11:
shichen = "巳时"
elif hour < 13:
shichen = "午时"
elif hour < 15:
shichen = "未时"
elif hour < 17:
shichen = "申时"
elif hour < 19:
shichen = "酉时"
elif hour < 21:
shichen = "戌时"
else:
shichen = "亥时"
最后,我们可以将时辰shichen打印出来:
print(shichen)
这样就可以将时间转换为时辰了。
### 回答3:
Python中可以使用`datetime`模块来将时间转换为时辰,具体步骤如下:
首先,需要导入datetime模块和calendar模块:
```python
import datetime
import calendar
```
接下来,使用`datetime.datetime.now()`函数获取当前时间,并将其保存在变量`now`中:
```python
now = datetime.datetime.now()
```
然后,使用`now.hour`和`now.minute`来获取当前时间的小时和分钟数,并保存在相应的变量中:
```python
hour = now.hour
minute = now.minute
```
接着,定义一个字典`hours`来存储每个时辰的起始小时数和终止小时数。根据时辰的定义,将24个小时平均分成12个时辰,每个时辰约为两个小时。
```python
hours = {
"子时": (0, 1),
"丑时": (1, 3),
"寅时": (3, 5),
"卯时": (5, 7),
"辰时": (7, 9),
"巳时": (9, 11),
"午时": (11, 13),
"未时": (13, 15),
"申时": (15, 17),
"酉时": (17, 19),
"戌时": (19, 21),
"亥时": (21, 23)
}
```
最后,根据当前的小时数和分钟数,通过遍历字典`hours`,找到对应的时辰并打印出来:
```python
for hour_name, (start_hour, end_hour) in hours.items():
if hour == end_hour or (start_hour < hour < end_hour) or (start_hour == hour and minute > 30):
print("当前时辰为:" + hour_name)
break
```
以上就是将时间转换为时辰的方法。通过获取当前的小时和分钟数,并根据定义好的时辰区间进行判断,就可以得到当前的时辰。
阅读全文
相关推荐
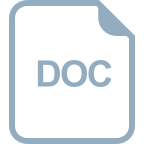
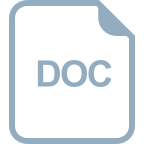
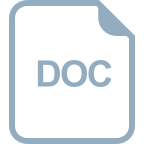
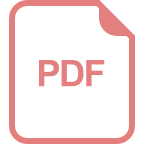
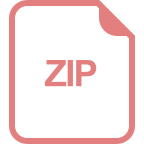
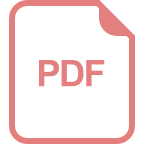
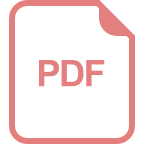
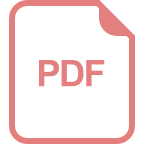
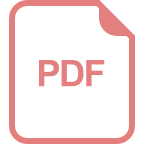
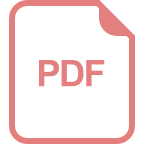
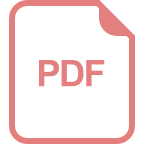
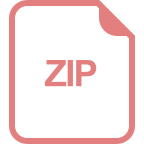
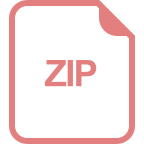