springboot + vue 验证码
时间: 2023-09-13 18:14:04 浏览: 156
您可以使用第三方库,如Google的reCAPTCHA,实现在Spring Boot和Vue中的验证码。
以下是使用reCAPTCHA的步骤:
1. 在Google reCAPTCHA网站上注册并获取网站密钥和秘密密钥。
2. 在Vue中,使用Google reCAPTCHA API添加reCAPTCHA小部件。您可以使用vue-recaptcha组件来实现它。
3. 在Spring Boot中,使用Google reCAPTCHA API验证用户提交的reCAPTCHA响应。您可以使用Google提供的Java客户端库来实现它。
以下是一个简单的示例:
Vue代码:
```
<template>
<div>
<vue-recaptcha
ref="recaptcha"
sitekey="YOUR_SITE_KEY"
@verify="onVerify"
></vue-recaptcha>
<button @click="submit">Submit</button>
</div>
</template>
<script>
import VueRecaptcha from 'vue-recaptcha';
export default {
components: {
VueRecaptcha,
},
methods: {
onVerify(response) {
this.recaptchaResponse = response;
},
submit() {
// send this.recaptchaResponse to server for verification
},
},
};
</script>
```
Spring Boot代码:
```
import com.google.api.client.googleapis.auth.oauth2.GoogleCredential;
import com.google.api.client.http.HttpRequest;
import com.google.api.client.http.HttpResponse;
import com.google.api.client.http.HttpTransport;
import com.google.api.client.http.javanet.NetHttpTransport;
import com.google.api.client.json.JsonObjectParser;
import com.google.api.client.json.jackson2.JacksonFactory;
import org.springframework.stereotype.Component;
import java.io.IOException;
import java.util.Arrays;
import java.util.Collections;
@Component
public class RecaptchaVerifier {
private static final String RECAPTCHA_VERIFY_URL = "https://www.google.com/recaptcha/api/siteverify";
private static final String SECRET_KEY = "YOUR_SECRET_KEY";
public boolean verify(String recaptchaResponse) throws IOException {
HttpTransport httpTransport = new NetHttpTransport();
GoogleCredential credential = new GoogleCredential.Builder()
.setTransport(httpTransport)
.setJsonFactory(new JacksonFactory())
.build();
HttpRequest request = httpTransport.createRequestFactory().buildPostRequest(
new com.google.api.client.http.GenericUrl(RECAPTCHA_VERIFY_URL),
new com.google.api.client.http.UrlEncodedContent(Collections.singletonMap("secret", SECRET_KEY))
.set("response", recaptchaResponse));
request.setParser(new JsonObjectParser(new JacksonFactory()));
HttpResponse response = request.execute();
RecaptchaVerificationResponse verificationResponse = response.parseAs(RecaptchaVerificationResponse.class);
return verificationResponse.success;
}
public static class RecaptchaVerificationResponse {
public boolean success;
public String[] error_codes;
}
}
```
请注意,您需要将YOUR_SITE_KEY和YOUR_SECRET_KEY替换为您在Google reCAPTCHA网站上注册的实际密钥。
阅读全文
相关推荐
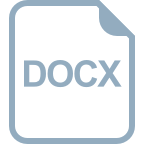
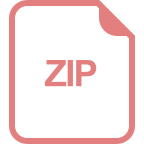

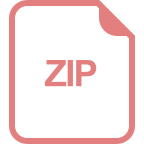


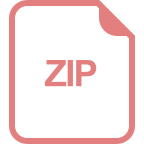
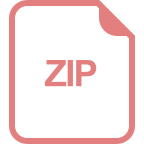
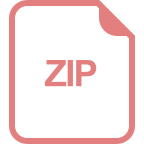
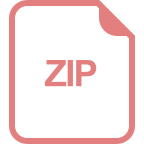
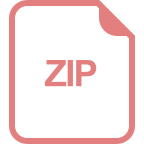
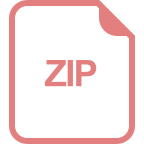
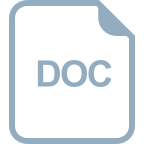
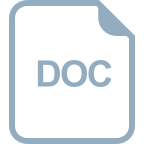
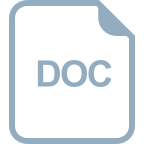


