springboot+vue验证码
时间: 2023-09-13 16:14:06 浏览: 105
这里提供一种使用Spring Boot和Vue.js实现验证码的方法:
1. 在Spring Boot中创建一个Controller,用于生成验证码图片和验证验证码。代码示例如下:
```java
@RestController
@RequestMapping("/api")
public class CaptchaController {
@Autowired
private DefaultKaptcha captchaProducer;
@GetMapping("/captcha")
public void captcha(HttpServletRequest request, HttpServletResponse response) throws Exception {
response.setHeader("Cache-Control", "no-store, no-cache");
response.setContentType("image/jpeg");
String captchaText = captchaProducer.createText();
request.getSession().setAttribute("captchaText", captchaText);
BufferedImage captchaImage = captchaProducer.createImage(captchaText);
ServletOutputStream out = response.getOutputStream();
ImageIO.write(captchaImage, "jpg", out);
out.flush();
out.close();
}
@PostMapping("/checkCaptcha")
public boolean checkCaptcha(HttpServletRequest request, @RequestBody String captcha) {
String captchaText = (String) request.getSession().getAttribute("captchaText");
return captchaText != null && captchaText.equalsIgnoreCase(captcha);
}
}
```
2. 在Vue.js中创建一个组件,用于显示验证码和验证。代码示例如下:
```vue
<template>
<div>
<img :src="captchaUrl" @click="refreshCaptcha" />
<input type="text" placeholder="请输入验证码" v-model="captcha" />
<button @click="checkCaptcha">验证</button>
</div>
</template>
<script>
export default {
data() {
return {
captchaUrl: '/api/captcha',
captcha: ''
}
},
methods: {
refreshCaptcha() {
this.captchaUrl = '/api/captcha?t=' + new Date().getTime()
},
checkCaptcha() {
this.$http.post('/api/checkCaptcha', this.captcha).then(response => {
if (response.data) {
alert('验证码正确')
} else {
alert('验证码错误')
this.refreshCaptcha()
}
})
}
}
}
</script>
```
3. 在页面中引入该组件即可:
```vue
<template>
<div>
<captcha />
</div>
</template>
<script>
import Captcha from './Captcha.vue'
export default {
components: {
Captcha
}
}
</script>
```
阅读全文
相关推荐

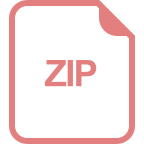


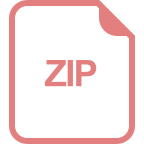
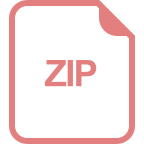
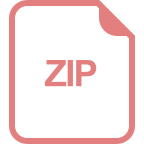
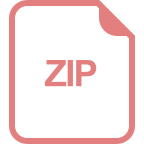
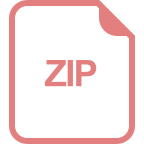
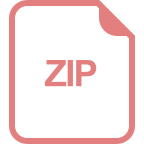
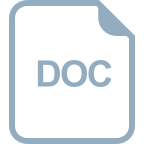
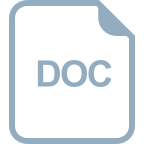
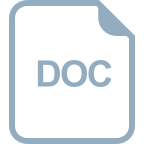


