请给出从人脸图像生成人脸UV纹理图的代码
时间: 2024-12-16 15:19:23 浏览: 11
人脸图像生成UV纹理图通常涉及到计算机图形学中的UV映射技术,这个过程可以分为两个步骤:首先获取人脸的关键点,然后将这些关键点投影到二维的UV坐标上形成纹理。由于这涉及到了深度学习和图形渲染的知识,这里我会提供一个简化的Python示例,使用一些库如OpenCV和trimesh,但这只是一个基础概念,实际应用可能会更复杂。
```python
import cv2
import numpy as np
from trimesh import Trimesh
# 1. 读取和处理人脸图像
def detect_face(image_path):
img = cv2.imread(image_path)
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
faces = face_cascade.detectMultiScale(img)
if len(faces) > 0:
x, y, w, h = faces[0]
return img[y:y+h, x:x+w]
else:
print("No face detected")
return None
# 2. 使用特征检测提取关键点
def extract_keypoints(face_image):
gray_img = cv2.cvtColor(face_image, cv2.COLOR_BGR2GRAY)
landmarks = face_recognition.face_landmarks(gray_img)
if landmarks:
return landmarks[0]['uv'] # 这里假设有 uv 字典返回关键点数据
else:
return []
# 3. 将关键点转换为UV坐标
def project_to_uv(landmarks, image_width, image_height):
uv_data = []
for point in landmarks:
u = (point[0] * image_width) / image.shape[1]
v = (point[1] * image_height) / image.shape[0]
uv_data.append((u, v))
return uv_data
# 示例
image_path = 'path/to/your/image.jpg'
face = detect_face(image_path)
keypoints = extract_keypoints(face)
uv_coordinates = project_to_uv(keypoints, face.shape[1], face.shape[0])
# 最后,你可能需要将这些UV坐标存储在一个适合的形式,比如Trimesh库,用于后续的纹理绘制或三维建模
mesh = Trimesh(vertices=..., faces=..., texture_uv=uv_coordinates)
```
阅读全文
相关推荐







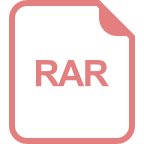


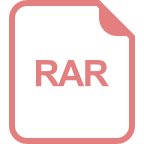
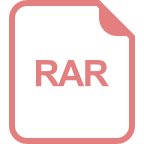





