python 粒子生长树代码
时间: 2023-09-18 18:13:53 浏览: 238
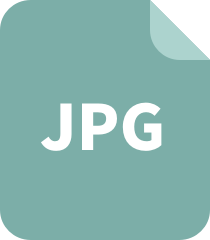
树的一个代码
以下是一个基于 Python 的粒子生长树代码示例,使用了 Pygame 库进行可视化:
```python
import pygame
import random
import math
pygame.init()
# 定义常量
WIDTH = 800
HEIGHT = 600
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
# 创建屏幕
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Particle Growth Tree")
# 定义粒子类
class Particle(pygame.sprite.Sprite):
def __init__(self, x, y, size):
super().__init__()
self.x = x
self.y = y
self.size = size
self.color = GREEN
self.speed = 2
self.angle = random.uniform(0, 360)
self.dx = math.cos(math.radians(self.angle)) * self.speed
self.dy = math.sin(math.radians(self.angle)) * self.speed
def update(self):
self.x += self.dx
self.y += self.dy
self.angle += random.uniform(-10, 10)
self.dx = math.cos(math.radians(self.angle)) * self.speed
self.dy = math.sin(math.radians(self.angle)) * self.speed
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.size)
# 定义树类
class Tree:
def __init__(self, x, y, size):
self.particles = pygame.sprite.Group()
self.x = x
self.y = y
self.size = size
self.color = BROWN
self.growing = True
self.max_particles = 500
# 添加树干
self.add_particle(x, y, size)
def add_particle(self, x, y, size):
particle = Particle(x, y, size)
self.particles.add(particle)
def grow(self):
if len(self.particles) < self.max_particles and self.growing:
for particle in self.particles:
if random.uniform(0, 1) > 0.8:
self.add_particle(particle.x, particle.y, particle.size * 0.8)
if len(self.particles) == self.max_particles:
self.growing = False
def update(self):
self.grow()
for particle in self.particles:
particle.update()
def draw(self):
for particle in self.particles:
particle.draw()
# 创建树
tree = Tree(WIDTH//2, HEIGHT, 20)
# 主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 更新和绘制树
tree.update()
screen.fill(WHITE)
tree.draw()
pygame.display.flip()
pygame.quit()
```
这是一个比较简单的示例,可以根据需要进行修改和扩展。
阅读全文
相关推荐



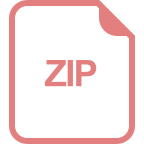
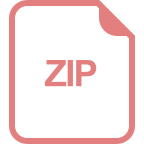
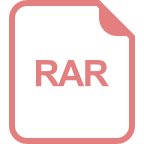
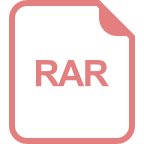
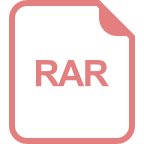
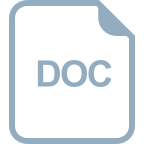
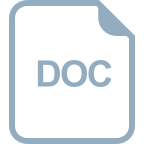
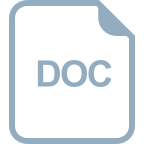
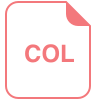
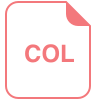
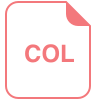
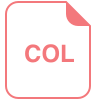
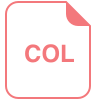
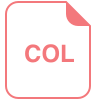