java 传输图片到前端的最佳做法例子
时间: 2024-10-03 08:04:09 浏览: 32
在Java应用中,将图片传送到前端通常涉及服务器端处理图片并将二进制数据作为响应发送给客户端。这里是一个简单的例子,使用Spring Boot框架和MultipartFile来处理文件上传:
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import javax.imageio.ImageIO;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class FileController {
@PostMapping("/upload-image")
public ResponseEntity<byte[]> uploadImage(@RequestParam("file") MultipartFile imageFile) {
if (imageFile.isEmpty()) {
return ResponseEntity.badRequest().body("No file selected.");
}
try {
// 检查文件类型是否支持
String allowedTypes = "image/jpeg, image/png";
if (!allowedTypes.contains(imageFile.getContentType())) {
return ResponseEntity.notAcceptable().body("Unsupported image type");
}
byte[] imageBytes = null;
// 将文件转换为字节数组
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ImageIO.write(imageFile.getBytes(), imageFile.getOriginalFilename().split("\\.")[-1], baos);
imageBytes = baos.toByteArray();
// 返回二进制数据作为响应
return ResponseEntity.ok()
.contentType(MediaType.IMAGE_JPEG) // 或者MediaType.IMAGE_PNG
.contentLength(imageBytes.length)
.body(imageBytes);
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Error processing image");
}
}
}
```
在前端,你可以使用axios等库来发起POST请求,并处理返回的数据:
```javascript
axios.post('/upload-image', {
file: formData // formData包含图片文件
}).then(response => {
let imageUrl = URL.createObjectURL(new Blob([response.data]));
// 使用imageUrl显示或保存图片
})
.catch(error => console.error(error));
```
阅读全文
相关推荐




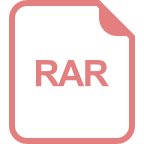


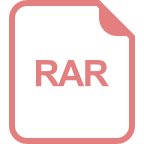
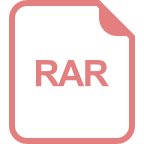
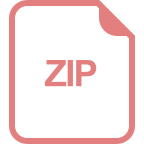

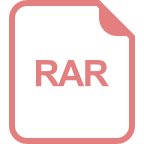
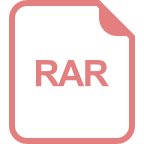

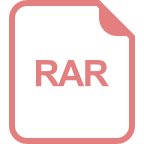
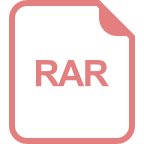
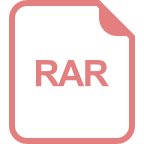

