遗传算法的程序编译python
时间: 2024-10-24 16:14:39 浏览: 20
遗传算法的程序编译并不涉及Python的编译过程,因为遗传算法本身是作为一种搜索优化方法,通常是在编程环境中作为算法实现的一部分,而不是单独作为一个可执行文件编译。在Python中,遗传算法的编写主要是通过定义类和函数来模拟自然选择的过程。
首先,要开始编写遗传算法来求解函数的最大值,你需要做以下几步[^1]:
1. 初始化种群(Population):创建一组随机生成的个体(代表可能的解决方案),每个个体由染色体(DNA编码)表示,可以是连续的数字或其他形式的变量。
```python
def initialize_population(size, problem):
# size 是种群大小,problem 是一个函数用于评估解的质量
return [random_solution(problem) for _ in range(size)]
# random_solution 函数负责生成随机解
```
2. 计算适应度(Fitness):给每个个体计算其适应度,即它们对应的目标函数值。
```python
def fitness(individual, problem):
return -problem(individual) # 负号是因为我们希望最大化函数值
# 求解函数的最大值,所以返回负值
fitness_of_population = [fitness(ind, problem) for ind in population]
```
3. 选择操作(Selection):从当前代中基于适应度选择父母。
```python
def selection(population, fitnesses, probability):
selected_indices = roulette_wheel_selection(fitnesses, probability)
return [population[i] for i in selected_indices]
# roulette_wheel_selection 依据适应度概率进行选择
```
4. 交叉(Crossover):在选定的父代之间进行基因重组。
```python
def crossover(parent1, parent2, crossover_rate):
if random.random() < crossover_rate:
child1, child2 = single_point_crossover(parent1, parent2)
else:
child1, child2 = parent1, parent2
return child1, child2
# single_point_crossover 实现单点交叉
```
5. 变异(Mutation):对子代进行微小变化以增加多样性。
```python
def mutate(child, mutation_rate):
if random.random() < mutation_rate:
mutated_child = mutate_one_gene(child)
else:
mutated_child = child
return mutated_child
# mutate_one_gene 实现单个基因变异
```
6. 重复这些步骤直到达到预定的迭代次数或找到满足条件的解。
最后,你可以将这些步骤组合成一个主循环,不断迭代直到找到最优解。
阅读全文
相关推荐







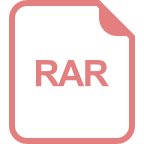










