用C++编写一个单链表,功能包含插入,查找,删除,合并,打印。放入主函数中运行
时间: 2024-11-13 13:28:18 浏览: 2
在C++中,我们可以创建一个简单的单链表结构,包括节点类`Node`用于存储数据和指向下一个节点的指针,以及链表类`LinkedList`实现插入、查找、删除和合并操作。以下是基本的实现:
```cpp
#include <iostream>
// 节点类
class Node {
public:
int data;
Node* next;
Node(int value) : data(value), next(nullptr) {}
};
// 链表类
class LinkedList {
private:
Node* head;
public:
// 构造函数
LinkedList() : head(nullptr) {}
// 插入节点
void insert(int value) {
Node* newNode = new Node(value);
if (head == nullptr) {
head = newNode;
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
// 查找节点
bool search(int value) {
Node* current = head;
while (current != nullptr) {
if (current->data == value) {
return true;
}
current = current->next;
}
return false;
}
// 删除节点
void remove(int value) {
if (head == nullptr) return;
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* prev = head;
Node* current = head->next;
while (current != nullptr && current->data != value) {
prev = current;
current = current->next;
}
if (current != nullptr) {
prev->next = current->next;
delete current;
}
}
// 打印链表
void printList() {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " -> ";
current = current->next;
}
std::cout << "nullptr" << std::endl;
}
// 合并两个有序链表
static LinkedList* merge(LinkedList* list1, LinkedList* list2) {
if (list1 == nullptr) return list2;
if (list2 == nullptr) return list1;
if (list1->search(list2->head->data)) {
return merge(list1->next, list2);
} else {
list1->insert(list2->head->data);
return merge(list1, list2->next);
}
}
};
int main() {
LinkedList list1, list2; // 创建两个链表
// 使用链表1进行示例操作
list1.insert(10);
list1.insert(20);
list1.insert(30);
// 打印链表1
std::cout << "Before merging:\n";
list1.printList();
// 合并链表
list2.insert(15);
list2.insert(25);
list2.insert(35);
LinkedList* mergedList = LinkedList::merge(&list1, &list2);
std::cout << "\nAfter merging:\n";
mergedList->printList();
return 0;
}
```
在这个例子中,我们实现了链表的基本操作,并在一个主函数中展示了如何使用这些功能。你可以根据需要修改或添加更多的功能。注意,这个例子假设链表头节点的数据是有序的。如果两个链表都无序,合并操作会变得复杂一些。
阅读全文
相关推荐
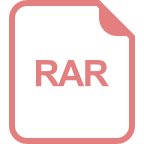
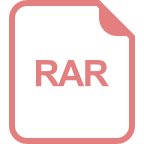
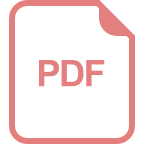















